防止sql注入参数化查询示例
时间: 2023-07-13 19:37:33 浏览: 106
假设我们有一个用户登录的功能,用户名和密码存储在数据库的 user 表中。我们可以使用参数化查询来防止 SQL 注入攻击,例如在 Python 中使用 MySQLdb 模块:
```python
import MySQLdb
# 连接数据库
conn = MySQLdb.connect(host='localhost', user='root', passwd='123456', db='test')
# 创建游标对象
cursor = conn.cursor()
# 执行参数化查询
username = "admin' or 1=1 -- " # 恶意输入
password = "123456"
sql = "SELECT * FROM user WHERE username=%s AND password=%s"
cursor.execute(sql, (username, password))
# 处理查询结果
result = cursor.fetchall()
if len(result) > 0:
print("登录成功")
else:
print("登录失败")
# 关闭游标和连接
cursor.close()
conn.close()
```
在上面的代码中,我们使用 `%s` 作为参数占位符,将 username 和 password 参数作为元组传递给 execute() 方法。这样,即使用户输入恶意的 SQL 代码,也不会对查询语句造成影响,因为这些恶意代码只会被当作参数值来处理。
相关问题
python防止sql注入的代码示例
为了防止SQL注入攻击,我们可以使用Python中的参数化查询。下面是一个Python防止SQL注入攻击的代码示例:
```
import mysql.connector
# 连接数据库
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="mydatabase"
)
# 创建游标对象
mycursor = mydb.cursor()
# 使用参数化查询防止SQL注入攻击
sql = "SELECT * FROM customers WHERE address = %s"
adr = ("Park Lane 38", )
mycursor.execute(sql, adr)
myresult = mycursor.fetchall()
for x in myresult:
print(x)
```
在上面的代码中,我们使用了参数化查询,将用户输入的值作为参数传递给SQL查询语句。这样可以防止SQL注入攻击,因为用户输入的值不会被解释为SQL语句的一部分,而是作为参数传递给查询。
阅读全文
相关推荐
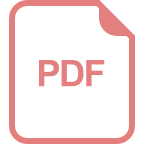
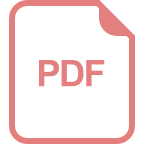
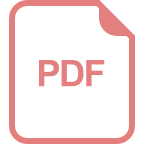
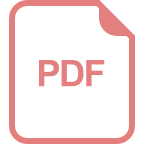
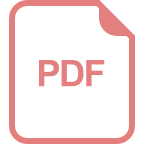
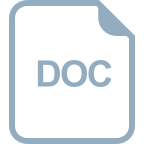
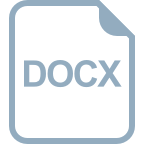
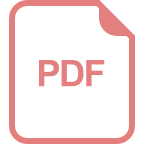
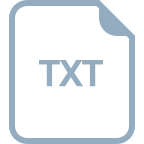
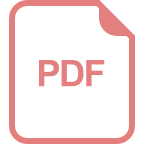
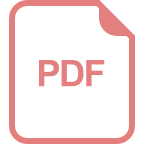
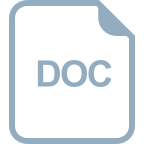
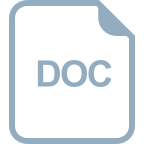
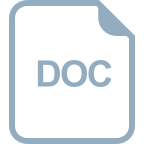
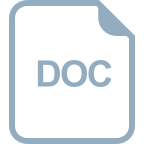
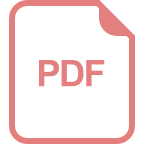