python实现xgboost回归贝叶斯调参
时间: 2023-06-21 11:06:08 浏览: 221
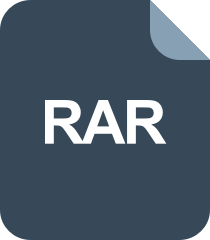
xgboost算法的python实现
XGBoost 是一种优秀的集成学习算法,它在分类和回归问题中都有着很好的表现。贝叶斯调参是一种基于贝叶斯优化的超参数优化技术,可以帮助我们更快地找到最优的超参数组合。下面是使用 Python 实现 XGBoost 回归贝叶斯调参的步骤:
1. 导入需要的库
```python
import xgboost as xgb
from sklearn.datasets import load_boston
from sklearn.model_selection import cross_val_score
from bayes_opt import BayesianOptimization
```
2. 加载数据集
```python
data = load_boston()
X = data['data']
y = data['target']
```
3. 定义 XGBoost 回归模型和目标函数
```python
def xgb_cv(max_depth, subsample, eta):
params = {
'eval_metric': 'rmse',
'max_depth': int(max_depth),
'subsample': subsample,
'eta': eta,
'seed': 42,
'nthread': -1,
}
cv_result = xgb.cv(params, xgb.DMatrix(X, y), num_boost_round=1000, nfold=5)
return -1.0 * cv_result['test-rmse-mean'].iloc[-1]
```
4. 定义超参数搜索空间
```python
xgb_bo = BayesianOptimization(
xgb_cv,
{'max_depth': (3, 7),
'subsample': (0.6, 1),
'eta': (0.01, 0.5)}
)
```
5. 进行贝叶斯调参优化
```python
xgb_bo.maximize(n_iter=10, init_points=10)
```
6. 输出最优超参数组合和最优目标函数值
```python
print(xgb_bo.max)
```
通过以上步骤,我们就可以使用 XGBoost 回归模型和贝叶斯调参技术来优化超参数,从而更好地拟合数据集。
阅读全文
相关推荐
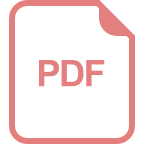


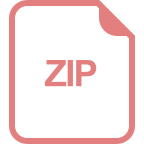
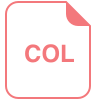
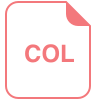
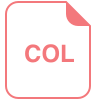
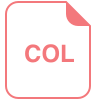
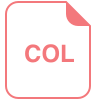
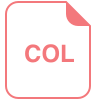
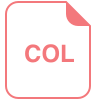
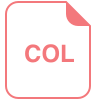
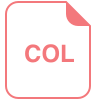


