如何用springboot 和RabbitMQ向指定用户发送通知
时间: 2023-07-26 19:08:06 浏览: 108
要向指定用户发送通知,需要使用RabbitMQ的Direct Exchange模式和Spring Boot的RabbitMQ支持。下面是基本步骤:
1. 首先,你需要在RabbitMQ中创建一个Direct Exchange,用于将消息路由到特定的队列。
2. 在Spring Boot应用程序中,你需要添加RabbitMQ依赖项,并配置RabbitMQ连接信息。
3. 创建一个消息发送者,该发送者将消息发送到指定的队列中。可以使用RabbitTemplate类来发送消息。
4. 创建一个消息接收者,该接收者从队列中接收消息并将其发送给指定的用户。可以使用@RabbitListener注释和@SendTo注释来实现这一点。
下面是一个简单的示例,演示如何使用Spring Boot和RabbitMQ向指定用户发送通知:
1. 创建一个Direct Exchange:
```java
@Configuration
public class RabbitMQConfig {
public static final String DIRECT_EXCHANGE = "directExchange";
@Bean
public DirectExchange directExchange() {
return new DirectExchange(DIRECT_EXCHANGE);
}
}
```
2. 配置RabbitMQ连接信息:
```properties
spring.rabbitmq.host=localhost
spring.rabbitmq.port=5672
spring.rabbitmq.username=guest
spring.rabbitmq.password=guest
```
3. 创建一个消息发送者:
```java
@Component
public class NotificationSender {
private final RabbitTemplate rabbitTemplate;
public NotificationSender(RabbitTemplate rabbitTemplate) {
this.rabbitTemplate = rabbitTemplate;
}
public void sendNotification(String userId, String message) {
rabbitTemplate.convertAndSend(RabbitMQConfig.DIRECT_EXCHANGE, userId, message);
}
}
```
4. 创建一个消息接收者:
```java
@Component
public class NotificationReceiver {
@RabbitListener(queues = "#{autoDeleteQueue1.name}")
@SendTo("#{headers['reply-to']}")
public String receiveNotification(String message) {
// 将消息发送给指定的用户
return "Notification received: " + message;
}
}
```
在这个示例中,消息接收者使用@RabbitListener注释来监听队列。当接收到消息时,它会将消息发送给指定的用户。@SendTo注释用于指定要将响应发送回的队列。
现在,你可以使用NotificationSender类向指定用户发送通知:
```java
@Service
public class NotificationService {
private final NotificationSender notificationSender;
public NotificationService(NotificationSender notificationSender) {
this.notificationSender = notificationSender;
}
public void sendNotification(String userId, String message) {
notificationSender.sendNotification(userId, message);
}
}
```
当你调用sendNotification方法时,它会将消息发送到RabbitMQ队列中,然后由消息接收者将消息发送给指定的用户。
希望这个示例对你有所帮助!
阅读全文
相关推荐
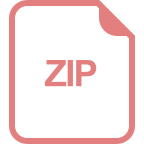



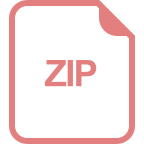
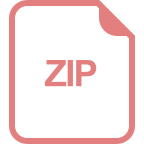
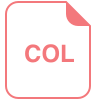
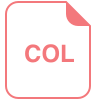



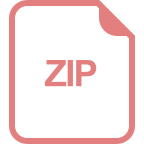
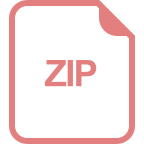
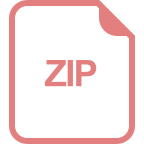