某个list<DTO>中,DTO中A字段顺序分别为2,3,4,5,6,7,12,15。用stream流的方式将DTO中的A字段顺序调整为1,2,3,4,5,6,7,8
时间: 2024-04-29 07:21:25 浏览: 136
可以使用以下代码实现:
```java
List<DTO> dtoList = ...; // 假设这是原始的 DTO 列表
Map<Integer, DTO> dtoMap = dtoList.stream()
.collect(Collectors.toMap(dto -> dto.getA(), dto -> dto));
List<DTO> sortedDTOList = IntStream.rangeClosed(1, 8)
.mapToObj(i -> dtoMap.get(i))
.filter(dto -> dto != null)
.collect(Collectors.toList());
```
代码的思路是先将 DTO 列表转换成一个 Map,其中 key 是 A 字段的值,value 是 DTO 对象本身。然后使用 IntStream 生成数字 1 到 8,依次从 Map 中获取对应的 DTO 对象,最后过滤掉 null 值并收集成一个新的 DTO 列表。这样就可以实现 A 字段的顺序调整了。
相关问题
java 用lambda表达式怎么遍历list<DTO> 并且筛选出dto中多个字段值为空对象并重新赋值
您好,对于您的问题,可以使用Java 8的Stream API和Lambda表达式来遍历list<DTO>并筛选出dto中多个字段值为空对象并重新赋值。具体实现可以参考以下代码:
```
list.stream().filter(dto -> dto.getField1() == null || dto.getField2() == null)
.forEach(dto -> {
if (dto.getField1() == null) {
dto.setField1(newValue1);
}
if (dto.getField2() == null) {
dto.setField2(newValue2);
}
});
```
其中,list为需要遍历的DTO列表,getField1和getField2为DTO中的两个字段,newValue1和newValue2为需要重新赋值的新值。使用filter方法筛选出字段值为空的DTO,然后使用forEach方法对每个符合条件的DTO进行重新赋值操作。
希望能够帮到您!
写一个用Ehcache读取2G的文件并缓存到临时文件中,最后以List<dto>的形式传出的demo
以下是一个使用 Ehcache 缓存大文件数据的示例代码。假设需要读取的文件为 `large_file.txt`,文件中每行包含两个字段,用空格分隔。
首先,需要在项目中添加 Ehcache 的依赖,并在 Ehcache 配置文件中定义一个缓存区,如下所示:
```xml
<ehcache:config xmlns:ehcache="http://www.ehcache.org/v3"
xmlns:jcache="http://www.ehcache.org/v3/jsr107">
<ehcache:cache alias="largeFileCache">
<ehcache:resources>
<ehcache:heap unit="MB">100</ehcache:heap>
</ehcache:resources>
</ehcache:cache>
</ehcache:config>
```
这里定义了一个名为 `largeFileCache` 的缓存区,并指定了最大堆内存为 100 MB。
然后,可以编写读取文件并缓存数据的代码:
```java
import org.ehcache.Cache;
import org.ehcache.CacheManager;
import org.ehcache.config.builders.CacheManagerBuilder;
import org.ehcache.config.builders.ExpiryPolicyBuilder;
import org.ehcache.config.builders.ResourcePoolsBuilder;
import org.ehcache.config.units.EntryUnit;
import org.ehcache.config.units.MemoryUnit;
import org.ehcache.expiry.ExpiryPolicy;
import org.ehcache.spi.loaderwriter.CacheLoaderWriter;
import org.ehcache.spi.loaderwriter.CacheLoaderWriterFactory;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.time.Duration;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.Callable;
public class LargeFileCacheDemo {
private static final String LARGE_FILE_PATH = "large_file.txt";
private static final String CACHE_ALIAS = "largeFileCache";
public static void main(String[] args) throws Exception {
CacheManager cacheManager = CacheManagerBuilder.newCacheManagerBuilder()
.withCache(CACHE_ALIAS, createCacheConfiguration())
.build(true);
Cache<String, List<DemoDto>> cache = cacheManager.getCache(CACHE_ALIAS, String.class, getListDtoType());
List<DemoDto> dtos = cache.get(LARGE_FILE_PATH);
if (dtos == null) {
loadFileAndCache(cache);
dtos = cache.get(LARGE_FILE_PATH);
}
System.out.println(dtos);
}
private static void loadFileAndCache(Cache<String, List<DemoDto>> cache) throws Exception {
Callable<List<DemoDto>> loader = () -> readAndParseFile();
CacheLoaderWriter<String, List<DemoDto>> loaderWriter = cache.getCacheLoaderWriterManager().getLoaderWriter(CACHE_ALIAS);
loaderWriter.write(LARGE_FILE_PATH, loader.call());
}
private static List<DemoDto> readAndParseFile() throws IOException {
List<DemoDto> dtos = new ArrayList<>();
try (BufferedReader reader = new BufferedReader(new FileReader(LARGE_FILE_PATH))) {
String line;
while ((line = reader.readLine()) != null) {
String[] fields = line.split(" ");
dtos.add(new DemoDto(fields[0], fields[1]));
}
}
return dtos;
}
private static CacheConfiguration<String, List<DemoDto>> createCacheConfiguration() {
return CacheConfigurationBuilder.newCacheConfigurationBuilder(
String.class,
getListDtoType(),
ResourcePoolsBuilder.newResourcePoolsBuilder()
.heap(100, EntryUnit.ENTRIES)
.offheap(1, MemoryUnit.GB)
.build())
.withExpiry(ExpiryPolicyBuilder.timeToLiveExpiration(Duration.ofMinutes(30)))
.withLoaderWriter(createCacheLoaderWriter())
.build();
}
private static CacheLoaderWriterFactory<String, List<DemoDto>> createCacheLoaderWriter() {
return new CacheLoaderWriterFactory<String, List<DemoDto>>() {
@Override
public CacheLoaderWriter<String, List<DemoDto>> createCacheLoaderWriter(String s, ClassLoader classLoader, CacheConfiguration<String, List<DemoDto>> cacheConfiguration) {
return new CacheLoaderWriter<String, List<DemoDto>>() {
@Override
public List<DemoDto> load(String key) throws Exception {
return readAndParseFile();
}
@Override
public void write(String key, List<DemoDto> value) throws Exception {
// Do nothing, as the data is already cached by the loader
}
@Override
public void delete(String key) throws Exception {
// Do nothing, as the data is not persisted
}
};
}
};
}
private static ParameterizedTypeReference<List<DemoDto>> getListDtoType() {
return new ParameterizedTypeReference<List<DemoDto>>() {};
}
private static class DemoDto {
private String field1;
private String field2;
public DemoDto(String field1, String field2) {
this.field1 = field1;
this.field2 = field2;
}
public String getField1() {
return field1;
}
public String getField2() {
return field2;
}
@Override
public String toString() {
return "DemoDto{" +
"field1='" + field1 + '\'' +
", field2='" + field2 + '\'' +
'}';
}
}
}
```
这个代码中,首先创建了一个 Ehcache 缓存管理器,并在其中定义了名为 `largeFileCache` 的缓存区。使用 `CacheManager.getCache()` 方法获取到 `largeFileCache` 缓存区的实例。然后,通过 `Cache.get()` 方法尝试从缓存区中获取数据。如果缓存中不存在数据,则使用 `CacheLoaderWriter.write()` 方法将数据写入缓存区。
`CacheLoaderWriter` 是一个接口,它定义了缓存装载器和写入器的行为。在这个示例中,使用 `CacheLoaderWriterFactory` 创建了一个 `CacheLoaderWriter` 的实例,它的 `load()` 方法读取文件并返回一个 DTO 对象列表,`write()` 方法不执行任何操作,因为数据已经被装载到缓存中,`delete()` 方法也不执行任何操作,因为数据并没有持久化。
`CacheConfigurationBuilder` 可以用来创建缓存配置对象,这里指定了缓存区的键和值类型,以及缓存区的容量和过期时间。
最后,定义了一个 `DemoDto` 类作为示例的 DTO 对象,它包含两个字符串字段。在 `readAndParseFile()` 方法中,读取文件并将每行解析为一个 `DemoDto` 对象,最终返回一个 `DemoDto` 对象列表。
注意,这个示例中使用了 Java 8 的 Lambda 表达式和函数式接口,需要在编译时指定 `-target 1.8` 或更高版本。
阅读全文
相关推荐
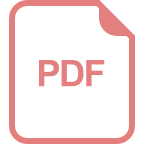
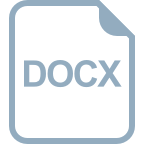













