使用SCALA语言,用NR算法实现分布式逻辑回归
时间: 2024-03-24 15:17:38 浏览: 73
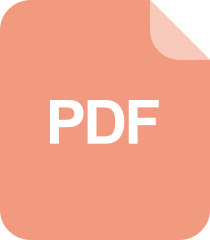
逻辑回归R语言算法

首先需要导入相关的库:
```scala
import org.apache.spark.mllib.classification.LogisticRegressionModel
import org.apache.spark.mllib.classification.LogisticRegressionWithSGD
import org.apache.spark.mllib.evaluation.BinaryClassificationMetrics
import org.apache.spark.mllib.regression.LabeledPoint
import org.apache.spark.{SparkConf, SparkContext}
```
接下来是实现逻辑回归的主要代码:
```scala
object DistributedLogisticRegression {
def main(args: Array[String]): Unit = {
val conf = new SparkConf().setAppName("DistributedLogisticRegression")
val sc = new SparkContext(conf)
// 加载数据集
val data = sc.textFile("data/mllib/sample_svm_data.txt")
val parsedData = data.map { line =>
val parts = line.split(' ')
LabeledPoint(parts(0).toDouble, org.apache.spark.mllib.linalg.Vectors.dense(parts.tail.map(_.toDouble)))
}.cache()
// 将数据集分为训练集和测试集
val splits = parsedData.randomSplit(Array(0.6, 0.4), seed = 11L)
val training = splits(0).cache()
val test = splits(1)
// 运行逻辑回归算法
val numIterations = 100
val model = LogisticRegressionWithSGD.train(training, numIterations)
// 对测试集进行预测
val predictionAndLabels = test.map { case LabeledPoint(label, features) =>
val prediction = model.predict(features)
(prediction, label)
}
// 计算模型的准确率、精确率、召回率、F1值
val metrics = new BinaryClassificationMetrics(predictionAndLabels)
val accuracy = predictionAndLabels.filter(r => r._1 == r._2).count.toDouble / test.count
val precision = metrics.precisionByThreshold.take(1)(0)._2
val recall = metrics.recallByThreshold.take(1)(0)._2
val f1Score = metrics.fMeasureByThreshold.take(1)(0)._2
// 输出结果
println(s"Accuracy = $accuracy")
println(s"Precision = $precision")
println(s"Recall = $recall")
println(s"F1 Score = $f1Score")
// 保存模型
model.save(sc, "model/distributedLogisticRegressionModel")
val sameModel = LogisticRegressionModel.load(sc, "model/distributedLogisticRegressionModel")
sc.stop()
}
}
```
首先,我们加载数据集并将其转换为LabeledPoint类型。然后,我们将数据集拆分为训练集和测试集,并运行逻辑回归算法。然后,我们对测试集进行预测,并计算模型的准确率、精确率、召回率和F1值。最后,我们将模型保存到本地磁盘并加载它。
注意,这里使用了Spark的机器学习库中的LogisticRegressionWithSGD类来实现逻辑回归。该类使用的是随机梯度下降算法。如果需要使用牛顿法实现逻辑回归,可以使用LogisticRegressionWithLBFGS类。
此外,如果需要使用正则化来避免过拟合,可以使用LogisticRegressionWithSGD类的setRegParam方法来设置正则化参数。
阅读全文
相关推荐




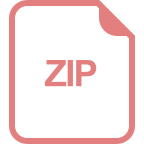
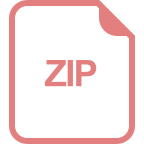
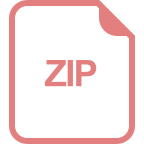
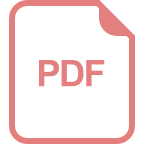
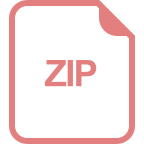
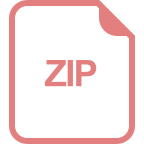
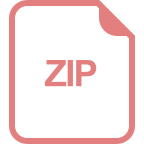
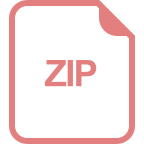
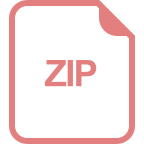
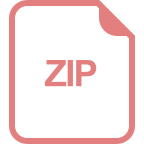
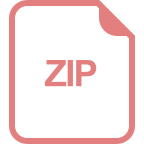