python怎么将列表写入text文件固定位置
时间: 2024-05-12 13:16:39 浏览: 73
您好!感谢您的问题。可以使用以下代码将列表写入 text 文件中的固定位置:
```python
# 要写入的列表
my_list = ["apple", "banana", "orange"]
# 打开文件并将列表写入文件中
with open("file.txt", "r+") as file:
# 将指针移动到固定位置
file.seek(10)
# 将列表转换为字符串并写入文件中
file.write(' '.join(my_list))
```
在这里,`r+` 模式用于在读取文件的同时写入数据。在这个例子中,它将指针移动到第 10 个位置,并将列表转换为字符串,然后将其写入文件中。
另外,请确保要以一种安全的方式存储包含任何敏感信息的文件,例如使用文件加密或访问控制列表(ACL)等技术。
相关问题
Python的fitz库文本固定显示在页面底部居中
要在fitz库中将文本固定显示在页面底部居中,你可以使用以下步骤:
1. 获取页面的大小和文本的大小。
2. 计算文本在页面中的位置。
3. 使用fitz库中的TextWriter对象将文本写入PDF文件中,并将其固定在计算出的位置。
下面是一个示例代码,其中文本为"Hello World!",页面大小为A4,字体为Helvetica,字号为12:
```python
import fitz
# 打开PDF文件并获取第一页
doc = fitz.open("example.pdf")
page = doc[0]
# 获取页面和文本的大小
page_width = page.MediaBoxSize[0]
page_height = page.MediaBoxSize[1]
text = "Hello World!"
text_width = page.getText("Hello World!", fontsize=12)[1][2] - page.getText("Hello World!", fontsize=12)[1][0]
# 计算文本在页面中的位置
x = (page_width - text_width) / 2
y = 50
# 创建TextWriter对象并将文本写入页面
writer = fitz.TextWriter(page, fontsize=12)
writer.horiz_align = "center"
writer.write_at(text, (x, y))
# 保存PDF文件
doc.save("example_with_text.pdf")
```
在这个示例中,我们首先打开了一个名为"example.pdf"的PDF文件,并获取了第一页。然后,我们获取了页面的大小和文本的大小,并计算了文本在页面中的位置。接下来,我们创建了一个TextWriter对象,并将其水平对齐方式设置为居中。最后,我们使用write_at方法将文本写入页面,并将其固定在计算出的位置。最后,我们保存了PDF文件并关闭了文档。
python写一个小程序,实现用按钮选择导入一个excel文件,生成固定样式的表格导出
要创建一个Python程序,利用`tkinter`库来构建用户界面,`pandas`处理Excel文件,并使用`openpyxl`或`xlsxwriter`库将数据写入新的Excel文件,你可以按照以下步骤操作:
```python
# 导入所需库
import tkinter as tk
from tkinter import filedialog
import pandas as pd
from openpyxl import Workbook
# 创建Tkinter窗口
root = tk.Tk()
root.title("Excel表格导入与导出")
# 定义函数:导入Excel文件
def import_excel():
file_path = filedialog.askopenfilename(
initialdir="/", title="选择Excel文件", filetypes=(("Excel files", "*.xlsx"), ("All files", "*.*"))
)
if file_path:
data = pd.read_excel(file_path)
# 这里可以根据需要对数据进行预处理
process_data(data)
# 定义函数:处理数据并导出到新Excel
def process_data(data):
# 指定样式等信息,这里简单示例,实际应用可根据需求定制
new_wb = Workbook()
ws = new_wb.active
ws['A1'] = '新表头'
ws.append(['数据', '描述'])
# 将数据写入新工作表
for i, row in data.iterrows():
ws.append(row)
# 保存新文件
save_file_path = filedialog.asksaveasfilename(
defaultextension=".xlsx",
initialfile="output.xlsx",
title="保存新Excel文件",
filetypes=(("Excel files", "*.xlsx"), ("All files", "*.*"))
)
if save_file_path:
new_wb.save(save_file_path)
print(f"已保存到 {save_file_path}")
# 添加导入和导出按钮
import_button = tk.Button(root, text="导入Excel", command=import_excel)
import_button.pack()
export_button = tk.Button(root, text="导出为Excel", state=tk.DISABLED) # 初始状态禁用
export_button.config(command=lambda: export_button["state"] = tk.NORMAL) # 点击后启用
export_button.pack()
# 运行主循环
root.mainloop()
```
当你运行这个程序,会弹出一个窗口,有两个按钮:一个用于导入Excel文件,另一个用于导出。点击导入按钮可以选择文件,然后处理数据;点击导出按钮,在导入文件后才能正常使用。
阅读全文
相关推荐
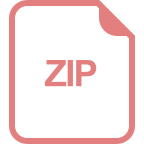
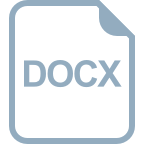
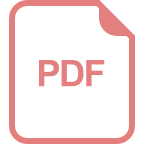
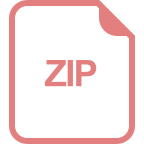
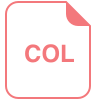
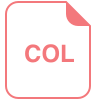
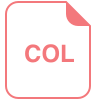
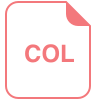
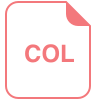
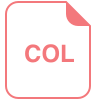
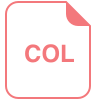
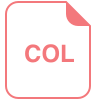
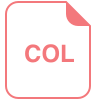
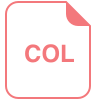
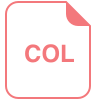
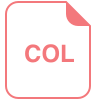