二叉树层序遍历c语言代码
时间: 2023-07-24 13:43:26 浏览: 58
下面是二叉树层序遍历的 C 语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_QUEUE_SIZE 100 // 队列最大容量
typedef struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
typedef struct Queue {
TreeNode *data[MAX_QUEUE_SIZE];
int front, rear;
} Queue;
// 初始化队列
void init(Queue *q) {
q->front = q->rear = 0;
}
// 判断队列是否为空
int is_empty(Queue *q) {
return q->front == q->rear;
}
// 入队
void enqueue(Queue *q, TreeNode *node) {
if ((q->rear + 1) % MAX_QUEUE_SIZE == q->front) {
printf("Queue is full.\n");
return;
}
q->data[q->rear] = node;
q->rear = (q->rear + 1) % MAX_QUEUE_SIZE;
}
// 出队
TreeNode *dequeue(Queue *q) {
if (is_empty(q)) {
printf("Queue is empty.\n");
return NULL;
}
TreeNode *node = q->data[q->front];
q->front = (q->front + 1) % MAX_QUEUE_SIZE;
return node;
}
// 层序遍历
void level_order(TreeNode *root) {
if (root == NULL) return;
Queue q;
init(&q);
enqueue(&q, root);
while (!is_empty(&q)) {
TreeNode *node = dequeue(&q);
printf("%d ", node->val);
if (node->left != NULL) enqueue(&q, node->left);
if (node->right != NULL) enqueue(&q, node->right);
}
}
int main() {
// 构建二叉树
TreeNode *root = (TreeNode *)malloc(sizeof(TreeNode));
root->val = 1;
root->left = (TreeNode *)malloc(sizeof(TreeNode));
root->left->val = 2;
root->right = (TreeNode *)malloc(sizeof(TreeNode));
root->right->val = 3;
root->left->left = NULL;
root->left->right = NULL;
root->right->left = (TreeNode *)malloc(sizeof(TreeNode));
root->right->left->val = 4;
root->right->right = (TreeNode *)malloc(sizeof(TreeNode));
root->right->right->val = 5;
root->right->left->left = NULL;
root->right->left->right = NULL;
root->right->right->left = NULL;
root->right->right->right = NULL;
// 层序遍历
level_order(root);
return 0;
}
```
输出结果为:1 2 3 4 5
相关推荐
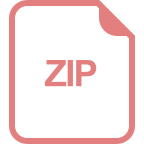
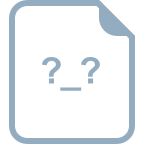
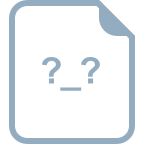














