二叉树的层序遍历c语言代码
时间: 2023-06-03 10:02:40 浏览: 66
以下是二叉树的层序遍历C语言代码:
```
#include <stdio.h>
#include <stdlib.h>
struct node {
int data;
struct node *left, *right;
};
void printLevelOrder(struct node* root)
{
// If tree is empty
if (root == NULL)
return;
// Create an empty queue for level order traversal
struct node* queue[1000];
int front = -1, rear = -1;
// Enqueue Root and initialize height
queue[++rear] = root;
while (1)
{
// nodeCount (queue size) indicates number of nodes
// at current level.
int nodeCount = rear-front;
if (nodeCount == 0)
break;
// Dequeue all nodes of current level and Enqueue
// all nodes of next level
while (nodeCount > 0)
{
struct node *node = queue[++front];
printf("%d ", node->data);
if (node->left != NULL)
queue[++rear] = node->left;
if (node->right != NULL)
queue[++rear] = node->right;
nodeCount--;
}
printf("\n");
}
}
struct node* newNode(int data)
{
struct node* node = (struct node*)malloc(sizeof(struct node));
node->data = data;
node->left = NULL;
node->right = NULL;
return node;
}
int main()
{
struct node* root = newNode(1);
root->left = newNode(2);
root->right = newNode(3);
root->left->left = newNode(4);
root->left->right = newNode(5);
printf("Level Order traversal of binary tree is \n");
printLevelOrder(root);
return 0;
}
```
相关推荐
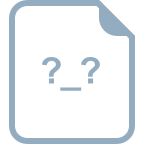
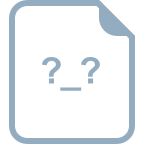
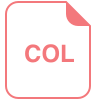
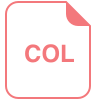
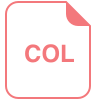
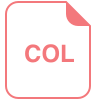
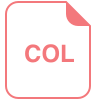









