二叉树层序遍历 C队列
时间: 2023-12-07 22:35:57 浏览: 56
二叉树的层序遍历是一种广度优先搜索算法,它按照从上到下、从左到右的顺序遍历二叉树的每个节点。而C队列是一种先进先出的数据结构,可以用来实现二叉树的层序遍历。具体实现方法是,先将根节点入队,然后依次取出队首节点,将其左右子节点入队,直到队列为空。这样就可以按照层序遍历的顺序遍历整个二叉树了。
下面是一个简单的C语言实现二叉树层序遍历的代码,其中使用了C队列:
```
#include <stdio.h>
#include <stdlib.h>
#include "tree.h" // 引用二叉树的头文件
#define MAX_QUEUE_SIZE 100 // 队列的最大长度
// 定义队列结构体
typedef struct {
BTNode* data[MAX_QUEUE_SIZE];
int front, rear;
} Queue;
// 初始化队列
void initQueue(Queue* q) {
q->front = q->rear = 0;
}
// 判断队列是否为空
bool isQueueEmpty(Queue* q) {
return q->front == q->rear;
}
// 判断队列是否已满
bool isQueueFull(Queue* q) {
return (q->rear + 1) % MAX_QUEUE_SIZE == q->front;
}
// 入队
void enqueue(Queue* q, BTNode* node) {
if (isQueueFull(q)) {
printf("Queue is full!\n");
exit(1);
}
q->data[q->rear] = node;
q->rear = (q->rear + 1) % MAX_QUEUE_SIZE;
}
// 出队
BTNode* dequeue(Queue* q) {
if (isQueueEmpty(q)) {
printf("Queue is empty!\n");
exit(1);
}
BTNode* node = q->data[q->front];
q->front = (q->front + 1) % MAX_QUEUE_SIZE;
return node;
}
// 二叉树的层序遍历
void TreeLevelOrder(BTNode* root) {
if (root == NULL) {
return;
}
Queue q;
initQueue(&q);
enqueue(&q, root);
while (!isQueueEmpty(&q)) {
BTNode* node = dequeue(&q);
printf("%c ", node->data);
if (node->left != NULL) {
enqueue(&q, node->left);
}
if (node->right != NULL) {
enqueue(&q, node->right);
}
}
}
```
相关推荐
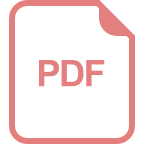
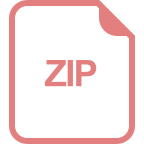
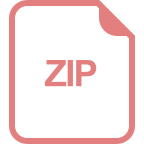
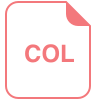
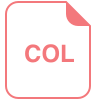
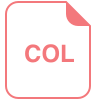
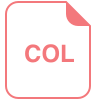
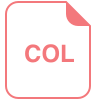









