restTemplate 发送 文件流
时间: 2024-01-05 09:04:32 浏览: 67
使用 RestTemplate 发送文件流可以通过 MultiValueMap 实现,以下是示例代码:
```java
import org.springframework.core.io.ByteArrayResource;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.RestTemplate;
public class RestTemplateSendFile {
public static void main(String[] args) {
byte[] byteArray = { 1, 2, 3, 4, 5 };
// 创建 RestTemplate 对象
RestTemplate restTemplate = new RestTemplate();
// 将 byte 数组封装为 ByteArrayResource 对象
ByteArrayResource resource = new ByteArrayResource(byteArray) {
@Override
public String getFilename() {
return "file.bin"; // 指定文件名
}
};
// 将文件添加到 MultiValueMap 中
MultiValueMap<String, Object> bodyMap = new LinkedMultiValueMap<>();
bodyMap.add("file", resource);
// 设置请求头
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.MULTIPART_FORM_DATA);
// 创建 HttpEntity 对象
HttpEntity<MultiValueMap<String, Object>> requestEntity = new HttpEntity<>(bodyMap, headers);
// 发送 POST 请求
String url = "http://example.com/upload";
ResponseEntity<String> response = restTemplate.postForEntity(url, requestEntity, String.class);
// 处理响应
// ...
}
}
```
在这个示例中,我们创建了一个长度为 5 的 byte 数组,并将它封装为一个 ByteArrayResource 对象,指定了文件名。然后,我们将这个对象添加到 MultiValueMap 中,以便在请求中发送。我们还设置了请求头,指定了请求体的类型为 Multipart。接着,我们创建了一个 HttpEntity 对象,将 MultiValueMap 对象作为请求体,并将其发送到指定的 URL。在响应中,我们可以获取服务器返回的内容并进行处理。
阅读全文
相关推荐
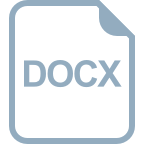










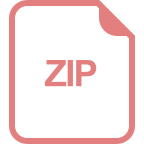






