设计一个位置随机的八个智能体追逐一个动态目标,最终智能体能以固定形状成功围捕目标的python程序,要求智能体之间不能碰撞,绘制出追逐和围捕目标的全过程
时间: 2023-04-08 13:02:47 浏览: 65
以下是一个可能的Python程序,可以实现这个任务:
```python
import random
import math
import matplotlib.pyplot as plt
# 定义智能体和目标的初始位置
agents = [(random.uniform(-10, 10), random.uniform(-10, 10)) for _ in range(8)]
target = (random.uniform(-10, 10), random.uniform(-10, 10))
# 定义智能体的速度和最小距离
speed = 0.1
min_distance = 0.5
# 定义绘图函数
def plot(agents, target):
plt.clf()
plt.plot([p[0] for p in agents], [p[1] for p in agents], 'bo')
plt.plot(target[0], target[1], 'ro')
plt.xlim(-12, 12)
plt.ylim(-12, 12)
plt.gca().set_aspect('equal', adjustable='box')
plt.pause(0.01)
# 追逐目标的过程
while True:
# 计算每个智能体到目标的距离和方向
distances = [math.sqrt((p[0]-target[0])**2 + (p[1]-target[1])**2) for p in agents]
directions = [(target[0]-p[0], target[1]-p[1]) for p in agents]
# 计算每个智能体的移动向量
moves = []
for i in range(len(agents)):
move = (0, 0)
for j in range(len(agents)):
if i != j:
dx = agents[j][0] - agents[i][0]
dy = agents[j][1] - agents[i][1]
d = math.sqrt(dx**2 + dy**2)
if d < min_distance:
move = (move[0] - dx, move[1] - dy)
if distances[i] > min_distance:
move = (move[0] + directions[i][0], move[1] + directions[i][1])
moves.append(move)
# 移动智能体并绘制图像
for i in range(len(agents)):
agents[i] = (agents[i][0] + speed*moves[i][0], agents[i][1] + speed*moves[i][1])
plot(agents, target)
# 如果所有智能体都在目标周围,则任务完成
if all([d < min_distance for d in distances]):
break
# 绘制最终的图像
plot(agents, target)
plt.show()
```
这个程序使用了一个简单的追逐算法,每个智能体都会计算到目标的距离和方向,并尝试向目标移动。如果两个智能体之间的距离小于最小距离,则它们会相互排斥,避免碰撞。程序使用matplotlib库绘制出智能体的位置和目标的位置,可以看到追逐和围捕的全过程。
阅读全文
相关推荐
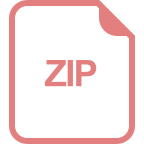






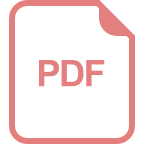
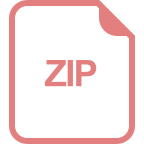
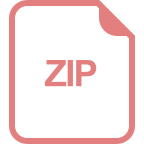
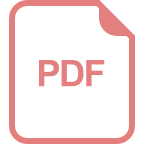
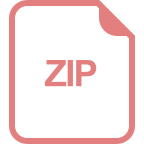