使用c语言1. 建立顺序栈,并在顺序栈上实现入栈和出栈操作(验证性内容)。
时间: 2024-02-03 18:12:47 浏览: 93
好的,以下是使用C语言实现顺序栈的代码,包括入栈和出栈操作:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 50
typedef struct {
int data[MAX_SIZE];
int top;
} Stack;
Stack* init() {
Stack* s = (Stack*)malloc(sizeof(Stack));
s->top = -1;
return s;
}
int is_empty(Stack* s) {
return s->top == -1;
}
int is_full(Stack* s) {
return s->top == MAX_SIZE - 1;
}
void push(Stack* s, int item) {
if (is_full(s)) {
printf("Stack is full, cannot push.\n");
return;
}
s->top++;
s->data[s->top] = item;
}
int pop(Stack* s) {
if (is_empty(s)) {
printf("Stack is empty, cannot pop.\n");
return -1;
}
int item = s->data[s->top];
s->top--;
return item;
}
int main() {
Stack* s = init();
push(s, 10);
push(s, 20);
push(s, 30);
printf("%d\n", pop(s));
printf("%d\n", pop(s));
printf("%d\n", pop(s));
printf("%d\n", pop(s));
free(s);
return 0;
}
```
这里我们使用了结构体来定义一个顺序栈,其中 `data` 数组存储栈中的元素,`top` 表示栈顶元素的下标,初始化为 `-1` 表示栈为空。`init()` 函数用于初始化一个栈,并返回栈的指针。`is_empty()` 和 `is_full()` 函数分别用于判断栈是否为空和是否已满。`push()` 函数用于将元素入栈,如果栈已满则输出提示信息。`pop()` 函数用于将栈顶元素出栈,并返回该元素的值,如果栈为空则输出提示信息。
在 `main()` 函数中,我们初始化了一个栈 `s`,并依次将元素 `10`、`20`、`30` 入栈,然后依次将栈中的元素出栈,并输出它们的值。最后,记得释放栈的内存空间。
希望对你有所帮助!
阅读全文
相关推荐
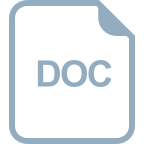
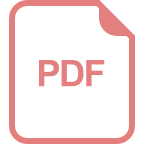
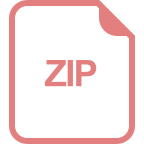
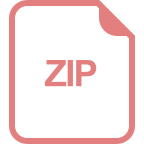
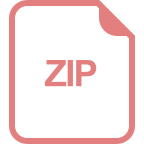
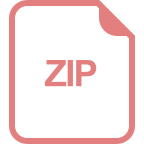
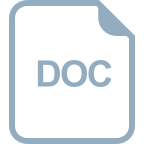
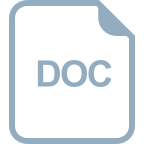
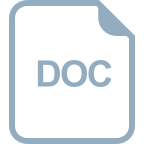
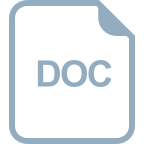
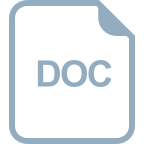
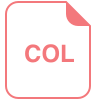
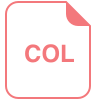


