python 实现子线程监听配置文件变换,如果服务停止那么子线程也停止
时间: 2024-01-31 14:02:42 浏览: 26
可以使用 Python 中的 threading 和 watchdog 库来实现子线程监听配置文件变化,并在服务停止时停止子线程。
示例代码如下:
```python
import threading
import time
from watchdog.observers import Observer
from watchdog.events import FileSystemEventHandler
class FileChangeHandler(FileSystemEventHandler):
def __init__(self, callback):
super().__init__()
self.callback = callback
def on_modified(self, event):
# 文件被修改时调用回调函数
if not event.is_directory:
self.callback()
class ConfigWatcher:
def __init__(self, config_path):
self.config_path = config_path
self.observer = Observer()
self.handler = FileChangeHandler(self._on_config_modified)
def start(self):
self.observer.schedule(self.handler, self.config_path, recursive=False)
self.observer.start()
def stop(self):
self.observer.stop()
self.observer.join()
def _on_config_modified(self):
# 配置文件被修改时的回调函数
print("Config file modified")
class MyService:
def __init__(self):
self.config_watcher = ConfigWatcher("/path/to/config")
def start(self):
# 启动配置文件监控器
self.config_watcher.start()
# 启动服务
while True:
print("Service is running")
time.sleep(1)
def stop(self):
# 停止配置文件监控器
self.config_watcher.stop()
# 停止服务
print("Service is stopped")
if __name__ == "__main__":
service = MyService()
# 启动服务
t = threading.Thread(target=service.start)
t.start()
# 等待用户输入停止服务
input("Press enter to stop the service\n")
# 停止服务
service.stop()
# 等待服务线程结束
t.join()
```
在上面的示例代码中,我们使用了 watchdog 库来监听配置文件的变化,并在文件被修改时调用回调函数 `_on_config_modified`。然后,我们使用 threading 库来创建一个子线程来运行服务的主循环,并在用户输入停止服务时调用服务的 `stop` 方法来停止服务。在 `stop` 方法中,我们停止配置文件监控器并等待服务线程结束。这样,当服务停止时,子线程也会停止。
相关推荐
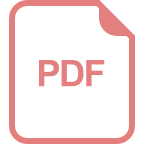














