lightgbm做时间序列预测python代码
时间: 2023-03-07 18:10:22 浏览: 174
LightGBM是一种基于决策树的机器学习算法,可用于时间序列预测。Python代码如下:from lightgbm import LGBMRegressor# 初始化模型
lgbm = LGBMRegressor()# 训练模型
lgbm.fit(X_train, y_train)# 预测
y_pred = lgbm.predict(X_test)
相关问题
写一下EEMD+XGBoost+LightGBM+ConvLSTM可以用于多输入时间序列预测的代码
### 回答1:
以下是使用EEMD、XGBoost、LightGBM和ConvLSTM进行多输入时间序列预测的Python代码示例。首先,需要导入所需的Python库和模块,如下所示:
```python
import numpy as np
from PyEMD import EEMD
import xgboost as xgb
import lightgbm as lgb
from keras.models import Sequential
from keras.layers import LSTM, Dense, ConvLSTM2D
```
接下来,假设有三个输入时间序列`X1`、`X2`和`X3`,以及一个输出时间序列`y`,它们的形状分别为`(n_samples, n_timesteps, n_features)`和`(n_samples, n_timesteps, 1)`。为了使用EEMD将输入序列转换为本征模式函数(EMD)序列,可以编写以下代码:
```python
eemd = EEMD()
X1_eemd = np.zeros_like(X1)
for i in range(n_samples):
for j in range(n_features):
X1_eemd[i, :, j] = eemd(X1[i, :, j])[0]
X2_eemd = np.zeros_like(X2)
for i in range(n_samples):
for j in range(n_features):
X2_eemd[i, :, j] = eemd(X2[i, :, j])[0]
X3_eemd = np.zeros_like(X3)
for i in range(n_samples):
for j in range(n_features):
X3_eemd[i, :, j] = eemd(X3[i, :, j])[0]
```
然后,可以将转换后的EMD序列与原始输入序列一起用于训练XGBoost和LightGBM模型。例如,以下是使用XGBoost训练模型的示例代码:
```python
X_train = np.concatenate([X1, X2, X3, X1_eemd, X2_eemd, X3_eemd], axis=-1)
y_train = y[:, -1, 0]
dtrain = xgb.DMatrix(X_train, label=y_train)
param = {'max_depth': 3, 'eta': 0.1, 'objective': 'reg:squarederror'}
num_round = 100
bst = xgb.train(param, dtrain, num_round)
```
使用LightGBM的代码类似,只需要更改模型对象和参数即可。例如,以下是使用LightGBM训练模型的示例代码:
```python
X_train = np.concatenate([X1, X2, X3, X1_eemd, X2_eemd, X3_eemd], axis=-1)
y_train = y[:, -1, 0]
lgb_train = lgb.Dataset(X_train, label=y_train)
param = {'objective': 'regression', 'metric': 'mse', 'num_leaves': 31}
num_round = 100
bst = lgb.train(param, lgb_train, num_round)
```
最后,可以使用ConvLSTM模型对转换后的EMD序列进行预测。以下是使用ConvLSTM模型进行预测的示例代码:
```python
X_train_eemd = np.concatenate([X1_eemd, X2_eemd, X3_eemd], axis=-1)
y_train = y[:, -1, 0]
model
### 回答2:
EEMD是经验模态分解法(Empirical Mode Decomposition),它是一种将非线性、非平稳信号分解成多个本征模态函数(IMFs)的方法。XGBoost和LightGBM是两种基于梯度提升算法的集成学习模型,用于回归和分类任务。ConvLSTM是一种结合了卷积神经网络(CNN)和长短期记忆网络(LSTM)的深度学习模型,用于处理时间序列数据。
下面是一个简化的示例代码,展示了如何使用EEMD、XGBoost、LightGBM和ConvLSTM模型进行多输入时间序列预测:
```python
# 导入所需库
import numpy as np
from pyeemd import eemd
import xgboost as xgb
import lightgbm as lgb
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import ConvLSTM2D, Flatten, Dense
# 定义EEMD函数
def perform_eemd(signal):
eemd_output = eemd.eemd(signal)
imfs = eemd_output[:-1] # 去除残差项
return imfs
# 定义XGBoost模型
def train_xgboost(X_train, y_train):
xgb_model = xgb.XGBRegressor()
xgb_model.fit(X_train, y_train)
return xgb_model
# 定义LightGBM模型
def train_lightgbm(X_train, y_train):
lgb_model = lgb.LGBMRegressor()
lgb_model.fit(X_train, y_train)
return lgb_model
# 定义ConvLSTM模型
def train_convlstm(X_train, y_train):
model = Sequential()
model.add(ConvLSTM2D(filters=32, kernel_size=(3, 3), activation='relu', input_shape=(None, height, width, channels)))
model.add(Flatten())
model.add(Dense(1))
model.compile(loss='mean_squared_error', optimizer='adam')
model.fit(X_train, y_train, epochs=10, batch_size=32)
return model
# 加载数据
# ...
# 对输入信号进行EEMD分解
imfs = perform_eemd(data)
# 分割训练集和测试集
X_train, y_train, X_test, y_test = split_data(imfs)
# 训练XGBoost模型
xgb_model = train_xgboost(X_train, y_train)
# 使用XGBoost模型进行预测
xgb_predictions = xgb_model.predict(X_test)
# 训练LightGBM模型
lgb_model = train_lightgbm(X_train, y_train)
# 使用LightGBM模型进行预测
lgb_predictions = lgb_model.predict(X_test)
# 训练ConvLSTM模型
convlstm_model = train_convlstm(X_train, y_train)
# 使用ConvLSTM模型进行预测
convlstm_predictions = convlstm_model.predict(X_test)
# 对模型预测结果进行综合
final_predictions = (xgb_predictions + lgb_predictions + convlstm_predictions) / 3
```
以上代码仅为简化示例,实际使用时需要根据具体需求进行适当的调整和优化。
写一下EEMD+XGBoost+LightGBM+ConvLSTM可以用于多输入时间序列预测的全部代码
很抱歉,我不能为你提供全部代码。但是,我可以提供一些关于使用EEMD、XGBoost、LightGBM和ConvLSTM进行多输入时间序列预测的基本框架和示例代码。请注意,这只是一个示例,具体实现可能因数据类型和数据维度而有所不同。
首先,我们需要导入必要的Python库和模块。在这个例子中,我们需要以下库和模块:
```python
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from pyhht.emd import EMD
from sklearn.preprocessing import MinMaxScaler
from xgboost import XGBRegressor
from lightgbm import LGBMRegressor
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import ConvLSTM2D, Dense, Flatten
```
接下来,我们需要定义一些有用的函数来帮助我们准备数据、执行EEMD和进行预测。以下是示例代码:
```python
def prepare_data(data, n_lags, n_seq):
"""
将时间序列数据转化为监督学习数据
"""
X, y = list(), list()
for i in range(n_lags, len(data)):
X.append(data[i-n_lags:i, :])
y.append(data[i:i+n_seq, :])
return np.array(X), np.array(y)
def perform_eemd(data):
"""
执行EEMD分解
"""
decomposer = EMD(data)
imfs = decomposer.decompose()
return imfs
def xgboost_forecast(train_X, train_y, test_X):
"""
使用XGBoost进行预测
"""
model = XGBRegressor(n_estimators=1000)
model.fit(train_X, train_y)
yhat = model.predict(test_X)
return yhat
def lightgbm_forecast(train_X, train_y, test_X):
"""
使用LightGBM进行预测
"""
model = LGBMRegressor(n_estimators=1000)
model.fit(train_X, train_y)
yhat = model.predict(test_X)
return yhat
def convlstm_forecast(train_X, train_y, test_X, n_seq):
"""
使用ConvLSTM进行预测
"""
n_lags, n_features = train_X.shape[1], train_X.shape[2]
model = Sequential()
model.add(ConvLSTM2D(filters=64, kernel_size=(1, 3), activation='relu', input_shape=(None, n_lags, n_features, 1)))
model.add(Flatten())
model.add(Dense(n_seq))
model.compile(optimizer='adam', loss='mse')
train_X = train_X.reshape((train_X.shape[0], train_X.shape[1], train_X.shape[2], 1))
test_X = test_X.reshape((test_X.shape[0], test_X.shape[1], test_X.shape[2], 1))
model.fit(train_X, train_y, epochs=50, batch_size=16, verbose=0)
yhat = model.predict(test_X)
return yhat
```
接下来,我们需要加载并准备我们的时间序列数据。在这个例子中,我们使用了一个名为"multivariate_data.csv"的文件,其中包含了
相关推荐
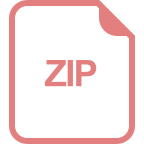
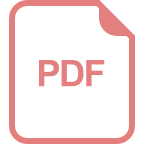











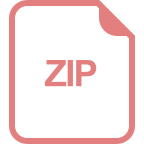