Springboot+html写一个增删改查源码
时间: 2023-06-11 17:04:29 浏览: 214
好的,以下是一个简单的SpringBoot + Thymeleaf 实现增删改查的示例代码:
(1)实体类:
```java
@Entity //告诉JPA这是一个实体类(和数据表映射的类)
public class Book {
@Id //这是主键
@GeneratedValue(strategy = GenerationType.IDENTITY) //自增主键
private Integer id;
private String name;
private String author;
//省略getter和setter方法
}
```
(2)控制层:
```java
@Controller
public class BookController {
@Autowired
private BookRepository bookRepository;
//查询所有图书列表
@GetMapping("/books")
public String list(Model model) {
model.addAttribute("books", bookRepository.findAll());
return "book/list";
}
//跳转到添加图书页面
@GetMapping("/book")
public String toAddPage() {
return "book/add";
}
//添加图书操作
@PostMapping("/book")
public String addBook(Book book) {
bookRepository.save(book);
return "redirect:/books";
}
//根据id查询图书
@GetMapping("/book/{id}")
public String toEditPage(@PathVariable("id") Integer id, Model model) {
Book book = bookRepository.getOne(id);
model.addAttribute("book", book);
return "book/edit";
}
//修改图书操作
@PutMapping("/book")
public String editBook(Book book) {
bookRepository.save(book);
return "redirect:/books";
}
//删除图书操作
@DeleteMapping("/book/{id}")
public String deleteBook(@PathVariable("id") Integer id) {
bookRepository.deleteById(id);
return "redirect:/books";
}
}
```
(3)视图层:
(3.1)图书列表页面(list.html):
```html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>图书列表</title>
</head>
<body>
<div align="center">
<h2>图书列表</h2>
<table border="1">
<thead>
<tr>
<th>ID</th>
<th>书名</th>
<th>作者</th>
</tr>
</thead>
<tbody>
<tr th:each="book : ${books}">
<td th:text="${book.id}"></td>
<td th:text="${book.name}"></td>
<td th:text="${book.author}"></td>
<td><a th:href="@{'/book/'+${book.id}}" th:text="编辑"></a></td>
<td><a th:href="@{'/book/'+${book.id}}" th:text="删除" method="DELETE"></a></td>
</tr>
</tbody>
</table>
<br>
<a href="/book">新增图书</a>
</div>
</body>
</html>
```
(3.2)添加图书页面(add.html):
```html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>新增图书</title>
</head>
<body>
<div align="center">
<h2>新增图书</h2>
<form th:action="@{/book}" th:object="${book}" method="POST">
<table>
<tr>
<td>书名</td>
<td><input type="text" th:field="*{name}" /></td>
</tr>
<tr>
<td>作者</td>
<td><input type="text" th:field="*{author}" /></td>
</tr>
<tr>
<td colspan="2" align="center">
<input type="submit" value="提交"/>
</td>
</tr>
</table>
</form>
</div>
</body>
</html>
```
(3.3)编辑图书页面(edit.html):
```html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>编辑图书</title>
</head>
<body>
<div align="center">
<h2>编辑图书</h2>
<form th:action="@{/book}" th:object="${book}" method="PUT">
<input type="hidden" th:field="*{id}" />
<table>
<tr>
<td>书名</td>
<td><input type="text" th:field="*{name}" /></td>
</tr>
<tr>
<td>作者</td>
<td><input type="text" th:field="*{author}" /></td>
</tr>
<tr>
<td colspan="2" align="center">
<input type="submit" value="保存"/>
</td>
</tr>
</table>
</form>
</div>
</body>
</html>
```
以上便是一个简单的 SpringBoot + Thymeleaf 实现增删改查的示例代码,希望能对您有所帮助。
阅读全文
相关推荐
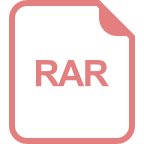















