31. 问题描述:通过python编程完成停车场收费模拟系统,具备其中一个功能,收费
时间: 2024-03-15 20:44:27 浏览: 69
好的,我可以帮你解答这个问题。停车场收费模拟系统可以分为两个部分:车辆进出管理和收费管理。其中,收费管理是整个系统的核心部分,需要考虑到不同时间段的不同收费标准,以及各种优惠政策的实现。
以下是一个简单的停车场收费模拟系统的代码示例,实现了基本的收费功能:
```python
import datetime
class ParkingLot:
def __init__(self, rate):
self.rate = rate
self.entries = {}
self.exits = {}
def enter(self, car_number):
now = datetime.datetime.now()
self.entries[car_number] = now
def exit(self, car_number):
now = datetime.datetime.now()
self.exits[car_number] = now
def get_duration(self, car_number):
entry_time = self.entries.get(car_number)
exit_time = self.exits.get(car_number)
if entry_time and exit_time:
duration = exit_time - entry_time
return duration.seconds // 60
else:
return None
def get_cost(self, car_number):
duration = self.get_duration(car_number)
if duration:
cost = duration * self.rate
return cost
else:
return None
if __name__ == '__main__':
parking_lot = ParkingLot(5) # 费率为每分钟5元
parking_lot.enter('京A12345')
parking_lot.exit('京A12345')
print(parking_lot.get_cost('京A12345'))
```
在上面的代码中,我们定义了一个 `ParkingLot` 类,它包含了停车场的收费率、车辆进出的记录、计算车辆停车时长和计算停车费用的方法。当车辆进入时,我们记录下进入时间;当车辆离开时,我们记录下离开时间。然后,我们可以通过 `get_duration` 方法计算出停车时长,再通过 `get_cost` 方法计算出停车费用。
在 `__main__` 函数中,我们创建了一个停车场实例,设置了停车费率为每分钟5元,模拟了一辆车进入并离开的过程,并打印出了停车费用。
当然,这只是一个简单的示例,实际的停车场收费系统可能需要考虑更多的情况,例如不同时间段的不同收费标准、会员优惠、免费停车时长等。但是,以上代码可以作为一个基础框架,帮助你进一步开发出更复杂的停车场收费模拟系统。
阅读全文
相关推荐
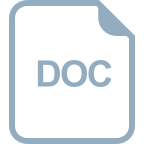
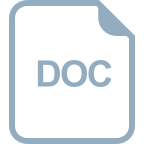
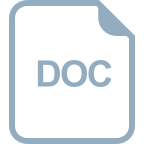
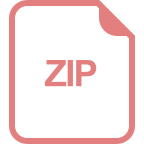
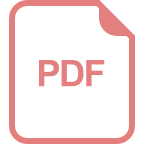
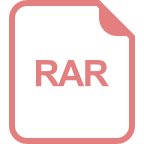
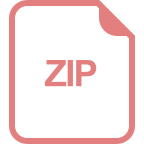
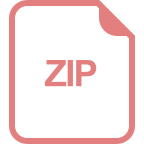
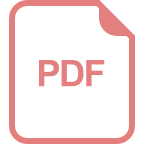
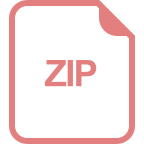
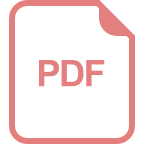
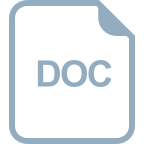
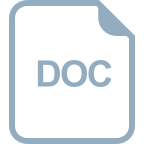
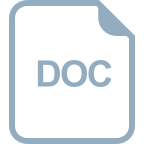
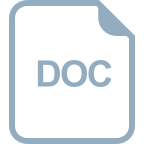
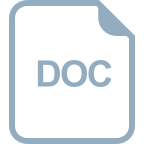
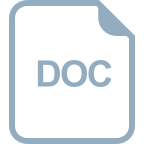

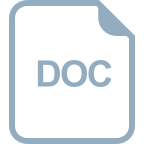
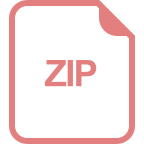