编写Java代码,实现hive自定义函数,将MySQL数据写入hive表
时间: 2023-08-15 17:27:37 浏览: 107
好的,下面是Java代码实现hive自定义函数,将MySQL数据写入hive表的过程:
```
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import org.apache.hadoop.hive.ql.exec.UDF;
import org.apache.hadoop.io.Text;
public class InsertMySQLDataToHive extends UDF {
public Text evaluate() {
String driver = "com.mysql.jdbc.Driver";
String url = "jdbc:mysql://localhost:3306/test";
String user = "root";
String password = "123456";
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
String tableName = "hive_table";
String mysqlTable = "mysql_table";
try {
Class.forName(driver);
conn = DriverManager.getConnection(url, user, password);
stmt = conn.createStatement();
rs = stmt.executeQuery("SELECT * FROM " + mysqlTable);
while (rs.next()) {
String column1 = rs.getString("column1");
String column2 = rs.getString("column2");
String column3 = rs.getString("column3");
String insertSql = "INSERT INTO TABLE " + tableName + " VALUES ('" + column1 + "','" + column2 + "','" + column3 + "')";
stmt.execute(insertSql);
}
return new Text("Insert MySQL data to Hive table successfully!");
} catch (ClassNotFoundException e) {
e.printStackTrace();
return new Text("ClassNotFoundException: " + e.getMessage());
} catch (SQLException e) {
e.printStackTrace();
return new Text("SQLException: " + e.getMessage());
} finally {
try {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
return new Text("SQLException: " + e.getMessage());
}
}
}
}
```
这个自定义函数连接到本地的MySQL数据库,从中读取数据并将其插入到Hive表中。在Hive中使用此自定义函数时,可以通过以下方式:
```
hive> ADD JAR /path/to/InsertMySQLDataToHive.jar;
hive> CREATE TEMPORARY FUNCTION insert_mysql_data_to_hive AS 'InsertMySQLDataToHive';
hive> SELECT insert_mysql_data_to_hive();
```
这将在Hive表中插入来自MySQL的数据,并在查询完成后返回“成功插入MySQL数据到Hive表!”。
阅读全文
相关推荐
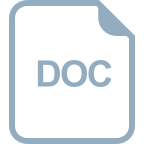
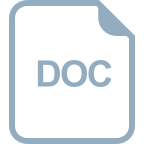
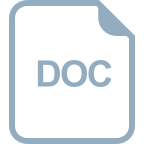

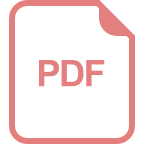
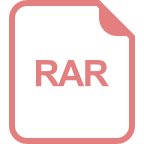
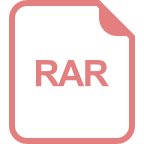
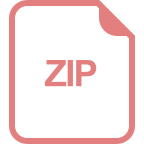
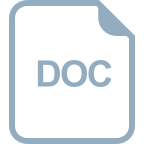
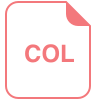
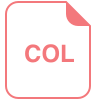
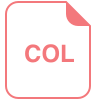
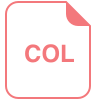
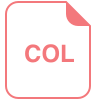
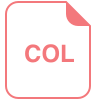




