怎么用C++写一个通讯录系统
时间: 2023-08-10 21:05:23 浏览: 40
以下是一个简单的通讯录系统的C++代码,它可以添加、查找、修改和删除联系人:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
struct Contact
{
string name;
string phone;
};
vector<Contact> contact_list;
void add_contact()
{
Contact c;
cout << "Enter name: ";
cin >> c.name;
cout << "Enter phone number: ";
cin >> c.phone;
contact_list.push_back(c);
cout << "Contact added successfully!" << endl;
}
void search_contact()
{
string name;
cout << "Enter name to search: ";
cin >> name;
for (int i = 0; i < contact_list.size(); i++)
{
if (contact_list[i].name == name)
{
cout << "Phone number for " << name << " is " << contact_list[i].phone << endl;
return;
}
}
cout << "Contact not found!" << endl;
}
void modify_contact()
{
string name;
cout << "Enter name to modify: ";
cin >> name;
for (int i = 0; i < contact_list.size(); i++)
{
if (contact_list[i].name == name)
{
cout << "Enter new phone number: ";
cin >> contact_list[i].phone;
cout << "Contact modified successfully!" << endl;
return;
}
}
cout << "Contact not found!" << endl;
}
void delete_contact()
{
string name;
cout << "Enter name to delete: ";
cin >> name;
for (int i = 0; i < contact_list.size(); i++)
{
if (contact_list[i].name == name)
{
contact_list.erase(contact_list.begin() + i);
cout << "Contact deleted successfully!" << endl;
return;
}
}
cout << "Contact not found!" << endl;
}
int main()
{
int choice;
while (true)
{
cout << endl << "Select an option:" << endl;
cout << "1. Add contact" << endl;
cout << "2. Search contact" << endl;
cout << "3. Modify contact" << endl;
cout << "4. Delete contact" << endl;
cout << "5. Exit" << endl;
cin >> choice;
switch (choice)
{
case 1:
add_contact();
break;
case 2:
search_contact();
break;
case 3:
modify_contact();
break;
case 4:
delete_contact();
break;
case 5:
return 0;
default:
cout << "Invalid choice!" << endl;
break;
}
}
}
```
在此示例中,我们使用一个结构体来表示联系人,并使用一个动态数组(vector)来保存联系人列表。通过使用循环和条件语句,我们可以在不同的选项中实现各种操作。
请注意,此代码仅供参考。如果您需要一个更复杂的通讯录系统,您可能需要更多的功能和错误处理。
相关推荐
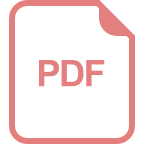
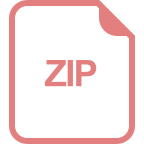














