用C++写一个通讯录管理系统,要求文件输入输出
时间: 2024-06-14 15:03:58 浏览: 203
以下是一个使用C++编写的通讯录管理系统,实现了通讯录联系人的添加、删除、查找、修改,以及通讯录的清空、备份和还原功能,并且支持文件输入输出:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
struct Contact {
string name;
string phone;
};
class AddressBook {
private:
vector<Contact> contacts;
public:
void addContact(const Contact& contact) {
contacts.push_back(contact);
}
void deleteContact(const string& name) {
for (auto it = contacts.begin(); it != contacts.end(); ++it) {
if (it->name == name) {
contacts.erase(it);
break;
}
}
}
Contact* findContact(const string& name) {
for (auto& contact : contacts) {
if (contact.name == name) {
return &contact;
}
}
return nullptr;
}
void modifyContact(const string& name, const string& newPhone) {
for (auto& contact : contacts) {
if (contact.name == name) {
contact.phone = newPhone;
break;
}
}
}
void clearAddressBook() {
contacts.clear();
}
void backupAddressBook(const string& filename) {
ofstream file(filename);
if (file.is_open()) {
for (const auto& contact : contacts) {
file << contact.name << "," << contact.phone << endl;
}
file.close();
cout << "通讯录已备份到文件:" << filename << endl;
} else {
cout << "无法打开文件:" << filename << endl;
}
}
void restoreAddressBook(const string& filename) {
ifstream file(filename);
if (file.is_open()) {
contacts.clear();
string line;
while (getline(file, line)) {
size_t pos = line.find(",");
if (pos != string::npos) {
string name = line.substr(0, pos);
string phone = line.substr(pos + 1);
contacts.push_back({name, phone});
}
}
file.close();
cout << "通讯录已从文件:" << filename << " 还原" << endl;
} else {
cout << "无法打开文件:" << filename << endl;
}
}
};
int main() {
AddressBook addressBook;
while (true) {
cout << "请选择操作:" << endl;
cout << "1. 添加联系人" << endl;
cout << "2. 删除联系人" << endl;
cout << "3. 查找联系人" << endl;
cout << "4. 修改联系人" << endl;
cout << "5. 清空通讯录" << endl;
cout << "6. 备份通讯录" << endl;
cout << "7. 还原通讯录" << endl;
cout << "8. 退出程序" << endl;
int choice;
cin >> choice;
if (choice == 1) {
cout << "请输入联系人姓名:";
string name;
cin >> name;
cout << "请输入联系人电话:";
string phone;
cin >> phone;
addressBook.addContact({name, phone});
cout << "联系人已添加" << endl;
} else if (choice == 2) {
cout << "请输入要删除的联系人姓名:";
string name;
cin >> name;
addressBook.deleteContact(name);
cout << "联系人已删除" << endl;
} else if (choice == 3) {
cout << "请输入要查找的联系人姓名:";
string name;
cin >> name;
Contact* contact = addressBook.findContact(name);
if (contact != nullptr) {
cout << "联系人姓名:" << contact->name << ",电话:" << contact->phone << endl;
} else {
cout << "未找到该联系人" << endl;
}
} else if (choice == 4) {
cout << "请输入要修改的联系人姓名:";
string name;
cin >> name;
cout << "请输入新的电话号码:";
string phone;
cin >> phone;
addressBook.modifyContact(name, phone);
cout << "联系人已修改" << endl;
} else if (choice == 5) {
addressBook.clearAddressBook();
cout << "通讯录已清空" << endl;
} else if (choice == 6) {
cout << "请输入备份文件名:";
string filename;
cin >> filename;
addressBook.backupAddressBook(filename);
} else if (choice == 7) {
cout << "请输入还原文件名:";
string filename;
cin >> filename;
addressBook.restoreAddressBook(filename);
} else if (choice == 8) {
break;
} else {
cout << "无效的选择,请重新输入" << endl;
}
}
return 0;
}
```
阅读全文
相关推荐
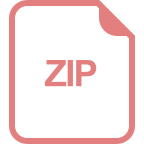
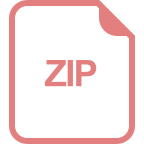
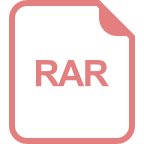
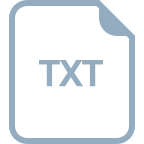
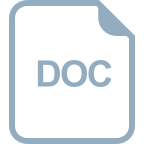
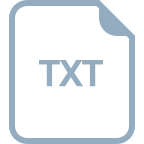
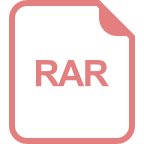
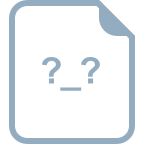
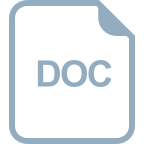
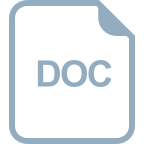
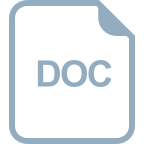
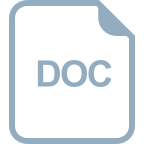
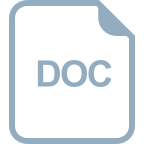
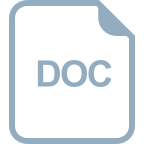
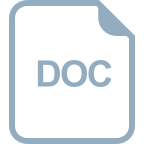
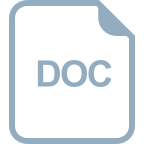
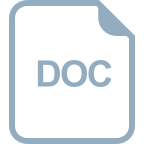
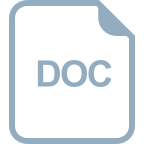