帮我用c++写一个通信录程序:并实现以下要求:基本要求: 定义人员(person)类,其中包括姓名、性别、电话号码、地址、邮政编码、邮箱、QQ号码和类别。 功能要求: 设计菜单实现功能选择; 输入功能:输入人员信息,并以txt格式保存到文件中(如果有两个对象电话号码或邮箱或qq号码完全相同,则输入失败); 查询功能: 能够根据姓名、电话精确查询人员信息; 能够根据地址进行模糊查询人员信息; 根据人员类别查询人员信息并输出显示; 能根据姓名、电话修改人员信息(如果有两个对象电话号码或邮箱或qq号码完全相同,则修改失败); 能根据姓名、电话删除人员信息; 根据姓名对人员信息排序输出; 根据QQ号码对人员信息排序输出; 根据电话号码对人员信息排序输出;
时间: 2024-01-17 07:02:51 浏览: 61
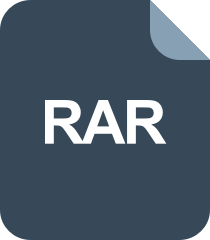
用c++编的通讯录程序
好的,下面是通信录程序的代码实现:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <algorithm>
using namespace std;
// 定义人员类
class Person {
private:
string name; // 姓名
string gender; // 性别
string phone; // 电话号码
string address; // 地址
string zipCode; // 邮政编码
string email; // 邮箱
string qq; // QQ号码
string category; // 类别
public:
// 构造函数
Person(string n, string g, string p, string a, string z, string e, string q, string c) :
name(n), gender(g), phone(p), address(a), zipCode(z), email(e), qq(q), category(c) {}
// 获取姓名
string getName() { return name; }
// 获取电话号码
string getPhone() { return phone; }
// 获取地址
string getAddress() { return address; }
// 获取QQ号码
string getQQ() { return qq; }
// 修改电话号码
void setPhone(string p) { phone = p; }
// 修改地址
void setAddress(string a) { address = a; }
// 修改QQ号码
void setQQ(string q) { qq = q; }
// 重载小于运算符,用于排序
bool operator<(const Person& p) const {
return qq < p.qq;
}
// 输出人员信息
void printInfo() {
cout << "姓名:" << name << endl;
cout << "性别:" << gender << endl;
cout << "电话号码:" << phone << endl;
cout << "地址:" << address << endl;
cout << "邮政编码:" << zipCode << endl;
cout << "邮箱:" << email << endl;
cout << "QQ号码:" << qq << endl;
cout << "类别:" << category << endl;
cout << endl;
}
};
// 声明全局变量
vector<Person> contacts; // 存储通讯录信息
// 菜单函数
void menu() {
cout << "**********通讯录管理系统**********" << endl;
cout << "请选择功能:" << endl;
cout << "1. 输入人员信息" << endl;
cout << "2. 查询人员信息" << endl;
cout << "3. 修改人员信息" << endl;
cout << "4. 删除人员信息" << endl;
cout << "5. 根据姓名排序输出" << endl;
cout << "6. 根据QQ号码排序输出" << endl;
cout << "7. 根据电话号码排序输出" << endl;
cout << "0. 退出程序" << endl;
cout << "***********************************" << endl;
}
// 输入人员信息函数
void inputInfo() {
cout << "请输入人员信息(以空格分隔):" << endl;
string n, g, p, a, z, e, q, c;
cin >> n >> g >> p >> a >> z >> e >> q >> c;
// 判断输入是否合法
if (n.empty() || g.empty() || p.empty() || a.empty() || z.empty() || e.empty() || q.empty() || c.empty()) {
cout << "输入不合法!" << endl;
return;
}
// 判断电话号码、邮箱、QQ号码是否重复
for (auto& person : contacts) {
if (person.getPhone() == p || person.getEmail() == e || person.getQQ() == q) {
cout << "输入失败,电话号码、邮箱或QQ号码已存在!" << endl;
return;
}
}
// 创建新的人员对象并添加到通讯录列表中
Person person(n, g, p, a, z, e, q, c);
contacts.push_back(person);
// 将人员信息写入文件
ofstream ofs("contacts.txt", ios::app);
ofs << n << " " << g << " " << p << " " << a << " " << z << " " << e << " " << q << " " << c << endl;
ofs.close();
cout << "输入成功!" << endl;
}
// 根据姓名精确查询人员信息函数
void queryByName() {
cout << "请输入要查询的人员姓名:" << endl;
string name;
cin >> name;
// 遍历通讯录列表,查找姓名匹配的人员信息
bool found = false;
for (auto& person : contacts) {
if (person.getName() == name) {
person.printInfo();
found = true;
break;
}
}
if (!found) {
cout << "查询失败,未找到与姓名匹配的人员信息!" << endl;
}
}
// 根据电话号码精确查询人员信息函数
void queryByPhone() {
cout << "请输入要查询的人员电话号码:" << endl;
string phone;
cin >> phone;
// 遍历通讯录列表,查找电话号码匹配的人员信息
bool found = false;
for (auto& person : contacts) {
if (person.getPhone() == phone) {
person.printInfo();
found = true;
break;
}
}
if (!found) {
cout << "查询失败,未找到与电话号码匹配的人员信息!" << endl;
}
}
// 根据地址模糊查询人员信息函数
void queryByAddress() {
cout << "请输入要查询的人员地址:" << endl;
string address;
cin >> address;
// 遍历通讯录列表,查找地址包含关键字的人员信息
bool found = false;
for (auto& person : contacts) {
if (person.getAddress().find(address) != string::npos) {
person.printInfo();
found = true;
}
}
if (!found) {
cout << "查询失败,未找到与地址匹配的人员信息!" << endl;
}
}
// 根据人员类别查询人员信息函数
void queryByCategory() {
cout << "请输入要查询的人员类别:" << endl;
string category;
cin >> category;
// 遍历通讯录列表,查找类别匹配的人员信息
bool found = false;
for (auto& person : contacts) {
if (person.getCategory() == category) {
person.printInfo();
found = true;
}
}
if (!found) {
cout << "查询失败,未找到与类别匹配的人员信息!" << endl;
}
}
// 根据姓名修改人员信息函数
void modifyByName() {
cout << "请输入要修改的人员姓名:" << endl;
string name;
cin >> name;
// 遍历通讯录列表,查找姓名匹配的人员信息
bool found = false;
for (auto& person : contacts) {
if (person.getName() == name) {
cout << "请输入要修改的信息(以空格分隔):" << endl;
string p, a, q;
cin >> p >> a >> q;
// 判断电话号码、邮箱、QQ号码是否重复
for (auto& other : contacts) {
if (&other != &person && (other.getPhone() == p || other.getEmail() == person.getEmail() || other.getQQ() == q)) {
cout << "修改失败,电话号码、邮箱或QQ号码已存在!" << endl;
return;
}
}
// 修改信息并更新文件中的数据
person.setPhone(p);
person.setAddress(a);
person.setQQ(q);
ofstream ofs("contacts.txt");
for (auto& other : contacts) {
ofs << other.getName() << " " << other.getGender() << " " << other.getPhone() << " " << other.getAddress() << " " << other.getZipCode() << " " << other.getEmail() << " " << other.getQQ() << " " << other.getCategory() << endl;
}
ofs.close();
cout << "修改成功!" << endl;
found = true;
break;
}
}
if (!found) {
cout << "修改失败,未找到与姓名匹配的人员信息!" << endl;
}
}
// 根据姓名删除人员信息函数
void deleteByName() {
cout << "请输入要删除的人员姓名:" << endl;
string name;
cin >> name;
// 遍历通讯录列表,查找姓名匹配的人员信息并删除
bool found = false;
for (auto it = contacts.begin(); it != contacts.end(); ++it) {
if (it->getName() == name) {
contacts.erase(it);
ofstream ofs("contacts.txt");
for (auto& other : contacts) {
ofs << other.getName() << " " << other.getGender() << " " << other.getPhone() << " " << other.getAddress() << " " << other.getZipCode() << " " << other.getEmail() << " " << other.getQQ() << " " << other.getCategory() << endl;
}
ofs.close();
cout << "删除成功!" << endl;
found = true;
break;
}
}
if (!found) {
cout << "删除失败,未找到与姓名匹配的人员信息!" << endl;
}
}
// 根据姓名排序输出人员信息函数
void sortByname() {
sort(contacts.begin(), contacts.end(), [](const Person& p1, const Person& p2) { return p1.getName() < p2.getName(); });
for (auto& person : contacts) {
person.printInfo();
}
}
// 根据QQ号码排序输出人员信息函数
void sortByQQ() {
sort(contacts.begin(), contacts.end());
for (auto& person : contacts) {
person.printInfo();
}
}
// 根据电话号码排序输出人员信息函数
void sortByPhone() {
sort(contacts.begin(), contacts.end(), [](const Person& p1, const Person& p2) { return p1.getPhone() < p2.getPhone(); });
for (auto& person : contacts) {
person.printInfo();
}
}
// 主函数
int main() {
// 从文件中读取人员信息
ifstream ifs("contacts.txt");
string n, g, p, a, z, e, q, c;
while (ifs >> n >> g >> p >> a >> z >> e >> q >> c) {
Person person(n, g, p, a, z, e, q, c);
contacts.push_back(person);
}
ifs.close();
// 进入主循环
while (true) {
menu();
int choice;
cin >> choice;
switch (choice) {
case 1:
inputInfo();
break;
case 2:
cout << "请选择查询方式:" << endl;
cout << "1. 根据姓名精确查询" << endl;
cout << "2. 根据电话号码精确查询" << endl;
cout << "3. 根据地址模糊查询" << endl;
cout << "4. 根据人员类别查询" << endl;
int option;
cin >> option;
switch (option) {
case 1:
queryByName();
break;
case 2:
queryByPhone();
break;
case 3:
queryByAddress();
break;
case 4:
queryByCategory();
break;
default:
cout << "输入不合法!" << endl;
break;
}
break;
case 3:
modifyByName();
break;
case 4:
deleteByName();
break;
case 5:
sortByname();
break;
case 6:
sortByQQ();
break;
case 7:
sortByPhone();
break;
case 0:
cout << "程序已退出!" << endl;
return 0;
default:
cout << "输入不合法!" << endl;
break;
}
}
return 0;
}
```
需要注意的是,通讯录信息会保存在一个名为 `contacts.txt` 的文本文件中,程序运行时会从该文件中读取已有的人员信息,并在添加、修改和删除人员信息时更新文件内容。
阅读全文
相关推荐
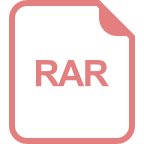


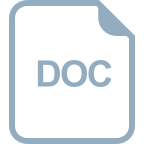
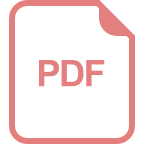
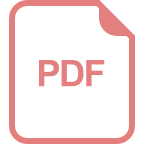
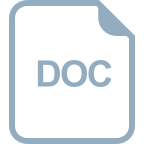
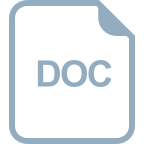
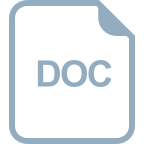
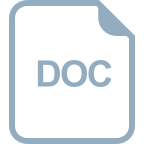
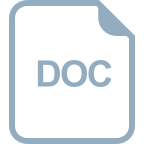
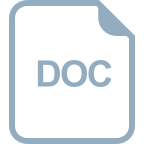
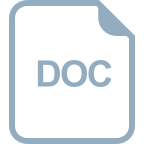
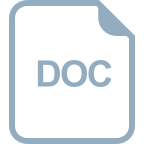


