像素坐标转相机坐标代码c++
时间: 2023-06-15 19:06:47 浏览: 33
以下是一个简单的 C++ 代码示例,将像素坐标转换为相机坐标:
```c++
cv::Mat camera_matrix; // 相机内参矩阵
cv::Mat distortion_coeffs; // 畸变系数
// 像素坐标
cv::Point2f pixel_point(100, 200);
// 相机坐标
cv::Mat camera_point(3, 1, cv::DataType<double>::type);
// 计算相机坐标
cv::Matx33d camera_matrix_mtx(camera_matrix);
cv::Matx<double, 5, 1> distortion_coeffs_mtx(distortion_coeffs);
cv::Matx31d pixel_point_mtx(pixel_point.x, pixel_point.y, 1.0);
cv::Matx33d inv_camera_matrix = camera_matrix_mtx.inv();
cv::Matx31d camera_point_mtx = inv_camera_matrix * pixel_point_mtx;
cv::Vec3d camera_point_vec(camera_point_mtx);
double r = sqrt(camera_point_vec[0] * camera_point_vec[0] + camera_point_vec[1] * camera_point_vec[1]);
double theta = atan(r);
double theta2 = theta * theta;
double theta4 = theta2 * theta2;
double theta6 = theta4 * theta2;
double theta8 = theta4 * theta4;
double radial_distortion = 1.0 + distortion_coeffs_mtx[0] * theta2 +
distortion_coeffs_mtx[1] * theta4 +
distortion_coeffs_mtx[4] * theta6;
camera_point_mtx[0] = camera_point_mtx[0] * radial_distortion + 2.0 * distortion_coeffs_mtx[2] * camera_point_vec[0] * camera_point_vec[1] +
distortion_coeffs_mtx[3] * (r2 + 2.0 * camera_point_vec[0] * camera_point_vec[0]);
camera_point_mtx[1] = camera_point_mtx[1] * radial_distortion + 2.0 * distortion_coeffs_mtx[3] * camera_point_vec[0] * camera_point_vec[1] +
distortion_coeffs_mtx[2] * (r2 + 2.0 * camera_point_vec[1] * camera_point_vec[1]);
camera_point_mtx[2] = 1.0;
camera_point = cv::Mat(camera_point_vec);
// 输出相机坐标
std::cout << "Camera Coordinates: " << camera_point << std::endl;
```
请注意,此代码假定您已经有相机内参矩阵和畸变系数,并且它使用 OpenCV 库来进行计算。如果您没有这些值,您需要使用相机校准的方法来获取它们。
相关推荐
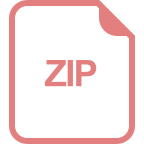

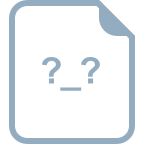
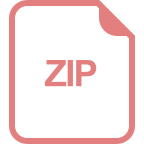
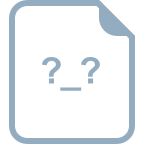
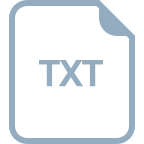
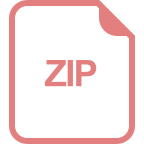
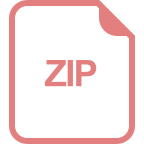
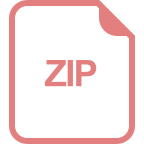
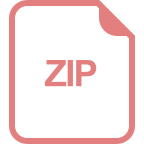
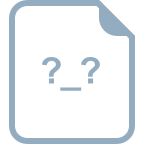
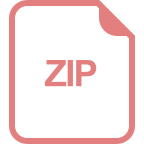
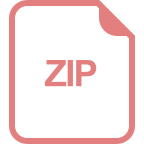
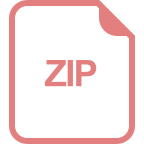