@JacksonXmlRootElement(localName = "BillHead") public class BillHead { //企业内部编号 @JacksonXmlProperty(localName = "CopListNo") private String CopListNo; //企业编号 @JacksonXmlProperty(localName = "CopNo") private String CopNo; } 获得Http客户端(可以理解为:你得先有一个浏览器;注意:实际上HttpClient与浏览器是不一样的) CloseableHttpClient httpClient = HttpClientBuilder.create().build(); // 创建Post请求 HttpPost httpPost = new HttpPost(WcsHttpUtil.httpUtil.agvs); // 设置ContentType(注:如果只是传普通参数的话,ContentType不一定非要用application/json) httpPost.setHeader("Content-Type", "application/json;charset=utf8"); // 响应模型 CloseableHttpResponse response = null; String jsonString = JSON.toJSONString(object); StringEntity entity = new StringEntity(jsonString, "UTF-8"); // post请求是将参数放在请求体里面传过去的;这里将entity放入post请求体中 httpPost.setEntity(entity); // 由客户端执行(发送)Post请求 response = httpClient.execute(httpPost); // 从响应模型中获取响应实体 HttpEntity responseEntity = response.getEntity(); 相应中获取响应实体数据为:HttpResponseProxy{HTTP/1.1 200 [Content-Type: application/xml, Content-Length: 120, Date: Tue, 23 May 2023 09:10:01 GMT, Keep-Alive: timeout=60, Connection: keep-alive] ResponseEntityProxy{[Content-Type: application/xml,Content-Length: 120,Chunked: false]}} 根据以上数据使用BillHead类接收
时间: 2023-11-28 15:48:39 浏览: 86
可以使用 Jackson XML 数据绑定工具将响应实体数据转换为 BillHead 类型的对象。
首先,需要在 pom.xml 文件中添加以下依赖:
```xml
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-xml</artifactId>
<version>2.12.4</version>
</dependency>
```
然后,可以使用以下代码将响应实体数据转换为 BillHead 对象:
```java
ObjectMapper mapper = new XmlMapper();
BillHead billHead = mapper.readValue(responseEntity.getContent(), BillHead.class);
```
其中,XmlMapper 是 Jackson XML 数据绑定工具的核心类,readValue() 方法可以将输入流中的 XML 数据转换为指定类型的 Java 对象。在这里,responseEntity.getContent() 方法返回一个输入流,表示响应实体的内容。最后,将转换后的 BillHead 对象返回即可。
相关问题
@JacksonXmlRootElement(localName = "BillHead") public class BillHead { //企业内部编号 @JacksonXmlProperty(localName = "CopListNo") private String CopListNo; //企业编号 @JacksonXmlProperty(localName = "CopNo") private String CopNo; } 获得Http客户端(可以理解为:你得先有一个浏览器;注意:实际上HttpClient与浏览器是不一样的) CloseableHttpClient httpClient = HttpClientBuilder.create().build(); // 创建Post请求 HttpPost httpPost = new HttpPost(WcsHttpUtil.httpUtil.agvs); // 设置ContentType(注:如果只是传普通参数的话,ContentType不一定非要用application/json) httpPost.setHeader("Content-Type", "application/json;charset=utf8"); // 响应模型 CloseableHttpResponse response = null; String jsonString = JSON.toJSONString(object); StringEntity entity = new StringEntity(jsonString, "UTF-8"); // post请求是将参数放在请求体里面传过去的;这里将entity放入post请求体中 httpPost.setEntity(entity); // 由客户端执行(发送)Post请求 response = httpClient.execute(httpPost); // 从响应模型中获取响应实体 HttpEntity responseEntity = response.getEntity(); 使用BillHead接收
可以使用以下代码来接收响应:
```
CloseableHttpResponse response = null;
try {
response = httpClient.execute(httpPost);
HttpEntity responseEntity = response.getEntity();
if (responseEntity != null) {
String responseString = EntityUtils.toString(responseEntity, "UTF-8");
BillHead billHead = JSON.parseObject(responseString, BillHead.class);
// 处理返回的BillHead对象
}
} finally {
if (response != null) {
response.close();
}
}
```
其中,`responseString` 是从响应实体中获取到的字符串,然后使用 `JSON.parseObject()` 方法将其转换为 `BillHead` 对象。最后你可以在使用 `billHead` 对象进行处理。
public class BillQueryResponse { @JacksonXmlProperty(localName = "InvtHead") private InvtHead invtHead; @JacksonXmlProperty(localName = "InvtListDetail") private InvtListDetail invtListDetail; @JacksonXmlProperty(localName = "InOutList") private InOutList inOutList; } org.springframework.http.converter.HttpMessageNotReadableException: JSON parse error: Cannot construct instance of com.emyiw.invsystem.entity.vo.BillQueryResponse (although at least one Creator exists): no String-argument constructor/factory method to deserialize from String value ('0'); nested exception is com.fasterxml.jackson.databind.exc.MismatchedInputException: Cannot construct instance of com.emyiw.invsystem.entity.vo.BillQueryResponse (although at least one Creator exists): no String-argument constructor/factory method to deserialize from String value ('0') at [Source: (PushbackInputStream); line: 43, column: 18] (through reference chain: com.emyiw.invsystem.entity.vo.Results["Data"]->java.util.ArrayList[1])解决以上报错信息
这个错误信息表明在使用JSON反序列化时,无法构造一个类型为`com.emyiw.invsystem.entity.vo.BillQueryResponse`的实例,因为该类中没有一个接受String类型参数的构造函数或工厂方法来从值“0”进行反序列化。但是,从你提供的代码来看,`BillQueryResponse`类似乎是一个XML序列化的类,并使用了`@JacksonXmlProperty`注解来指定XML元素名称。因此,你需要使用XML反序列化器来反序列化该对象,而不是使用JSON反序列化器。
你可以在`com.fasterxml.jackson.dataformat.xml.XmlMapper`类中使用`readValue`方法来反序列化XML字符串。例如:
```java
XmlMapper xmlMapper = new XmlMapper();
BillQueryResponse response = xmlMapper.readValue(xmlString, BillQueryResponse.class);
```
这样就可以正确地反序列化`BillQueryResponse`对象了。
阅读全文
相关推荐
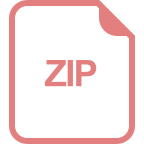
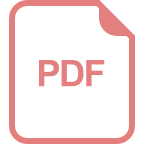
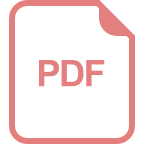













