boost threadpool 中bool wait(xtime const & timestamp, size_t task_threshold = 0) const函数的用法
时间: 2024-03-06 12:50:42 浏览: 21
`bool wait(xtime const & timestamp, size_t task_threshold = 0) const`是boost.threadpool中的一个函数,用于等待线程池中的任务完成。该函数接受两个参数:`xtime const & timestamp`和`size_t task_threshold`。
其中,`xtime const & timestamp`是一个xtime结构体,表示等待的时间戳。该结构体的定义如下:
```
struct xtime {
long sec; // 秒
long nsec; // 纳秒
};
```
`size_t task_threshold`表示任务阈值,当线程池中的任务数量小于该值时,`wait()`函数返回false。
`wait()`函数会阻塞当前线程,直到所有任务都已经完成或者等待时间戳已经到达。如果所有任务都已经完成,函数返回true;如果等待时间戳已经到达,但是还有未完成的任务,函数返回false。
下面是一个示例代码,其中使用`wait()`函数等待任务完成:
```
#include <iostream>
#include <boost/threadpool.hpp>
using namespace std;
using namespace boost::threadpool;
int main()
{
// 创建一个线程池对象,参数为线程池中线程数量
threadpool pool(4);
// 添加任务
for (int i = 0; i < 10; ++i)
{
pool.add_task([]()
{
cout << "Task " << boost::this_thread::get_id() << " is running" << endl;
boost::this_thread::sleep_for(boost::chrono::milliseconds(1000)); // 延时等待1秒
cout << "Task " << boost::this_thread::get_id() << " is done" << endl;
});
}
// 等待任务完成,并在2秒后回收线程池中的线程资源
xtime xt;
xtime_get(&xt, TIME_UTC);
xt.sec += 2; // 2秒后超时
bool success = pool.wait(xt);
if (success)
{
cout << "All tasks have been completed!" << endl;
}
else
{
cout << "Timeout! Some tasks are still running." << endl;
}
// 释放线程池
pool.join();
return 0;
}
```
在上面的示例代码中,我们使用`xtime_get()`函数获取当前时间,并将等待时间戳设置为当前时间+2秒。在调用`wait()`函数时,如果线程池中的所有任务都已经完成,函数会返回true,并输出"All tasks have been completed!";如果等待时间戳已经到达,但是还有未完成的任务,函数会返回false,并输出"Timeout! Some tasks are still running."。最后,我们释放线程池中的线程资源。
相关推荐
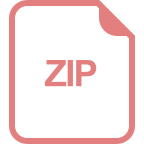
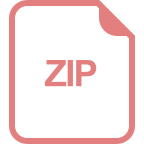














