bp神经网络minst分类
时间: 2023-10-07 08:04:20 浏览: 98
MNIST数据集是一个手写数字图像数据集,其中包含60,000个训练样本和10,000个测试样本。每个图像都是28x28像素,并且灰度级别为0到255。BP神经网络是一种常用的人工神经网络,可以用于分类问题。
以下是使用Python和TensorFlow实现BP神经网络对MNIST数据集进行分类的简要步骤:
1. 导入必要的库
```python
import tensorflow as tf
from tensorflow.examples.tutorials.mnist import input_data
```
2. 加载MNIST数据集
```python
mnist = input_data.read_data_sets("MNIST_data/", one_hot=True)
```
3. 定义输入和输出占位符
```python
x = tf.placeholder(tf.float32, [None, 784])
y_ = tf.placeholder(tf.float32, [None, 10])
```
4. 定义权重和偏置变量
```python
W = tf.Variable(tf.zeros([784, 10]))
b = tf.Variable(tf.zeros([10]))
```
5. 定义模型
```python
y = tf.nn.softmax(tf.matmul(x, W) + b)
```
6. 定义损失函数
```python
cross_entropy = tf.reduce_mean(-tf.reduce_sum(y_ * tf.log(y), reduction_indices=[1]))
```
7. 定义优化器
```python
train_step = tf.train.GradientDescentOptimizer(0.5).minimize(cross_entropy)
```
8. 训练模型
```python
sess = tf.InteractiveSession()
tf.global_variables_initializer().run()
for _ in range(1000):
batch_xs, batch_ys = mnist.train.next_batch(100)
sess.run(train_step, feed_dict={x: batch_xs, y_: batch_ys})
```
9. 测试模型
```python
correct_prediction = tf.equal(tf.argmax(y,1), tf.argmax(y_,1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
print(sess.run(accuracy, feed_dict={x: mnist.test.images, y_: mnist.test.labels}))
```
完整代码如下:
```python
import tensorflow as tf
from tensorflow.examples.tutorials.mnist import input_data
# 加载MNIST数据集
mnist = input_data.read_data_sets("MNIST_data/", one_hot=True)
# 定义输入和输出占位符
x = tf.placeholder(tf.float32, [None, 784])
y_ = tf.placeholder(tf.float32, [None, 10])
# 定义权重和偏置变量
W = tf.Variable(tf.zeros([784, 10]))
b = tf.Variable(tf.zeros([10]))
# 定义模型
y = tf.nn.softmax(tf.matmul(x, W) + b)
# 定义损失函数
cross_entropy = tf.reduce_mean(-tf.reduce_sum(y_ * tf.log(y), reduction_indices=[1]))
# 定义优化器
train_step = tf.train.GradientDescentOptimizer(0.5).minimize(cross_entropy)
# 训练模型
sess = tf.InteractiveSession()
tf.global_variables_initializer().run()
for _ in range(1000):
batch_xs, batch_ys = mnist.train.next_batch(100)
sess.run(train_step, feed_dict={x: batch_xs, y_: batch_ys})
# 测试模型
correct_prediction = tf.equal(tf.argmax(y,1), tf.argmax(y_,1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
print(sess.run(accuracy, feed_dict={x: mnist.test.images, y_: mnist.test.labels}))
```
执行上述代码,即可实现BP神经网络对MNIST数据集的分类。
阅读全文
相关推荐



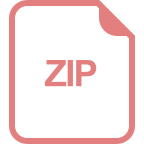
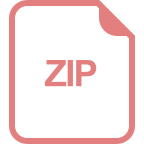
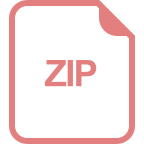
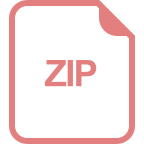
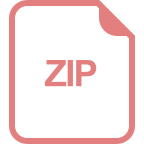
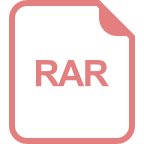


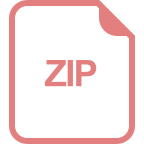
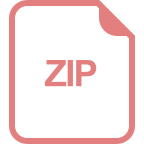
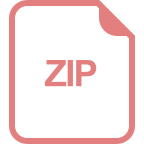
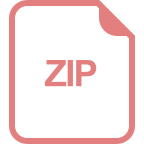