使用php 写一个redis控制类
时间: 2024-01-19 13:04:06 浏览: 33
好的,以下是一个简单的 Redis 控制类示例,基于 PHP Redis 扩展库实现:
```php
<?php
// Redis 控制类
class RedisControl {
private $redis;
private $host;
private $port;
private $password;
private $timeout;
// 构造函数
public function __construct($host='127.0.0.1', $port=6379, $password='', $timeout=0) {
$this->host = $host;
$this->port = $port;
$this->password = $password;
$this->timeout = $timeout;
$this->redis = new Redis();
$this->redis->connect($host, $port, $timeout);
if (!empty($password)) {
$this->redis->auth($password);
}
}
// 获取 Redis 对象
public function getRedis() {
return $this->redis;
}
// 设置缓存
public function set($key, $value, $expire=0) {
if ($expire > 0) {
return $this->redis->setex($key, $expire, $value);
} else {
return $this->redis->set($key, $value);
}
}
// 获取缓存
public function get($key) {
return $this->redis->get($key);
}
// 删除缓存
public function delete($key) {
return $this->redis->delete($key);
}
// 自增
public function incr($key) {
return $this->redis->incr($key);
}
// 自减
public function decr($key) {
return $this->redis->decr($key);
}
// 获取所有缓存的 key
public function keys($pattern) {
return $this->redis->keys($pattern);
}
// 清空缓存
public function flush() {
return $this->redis->flushDB();
}
// 关闭连接
public function close() {
return $this->redis->close();
}
// 析构函数
public function __destruct() {
$this->close();
}
}
```
在使用时,您可以先实例化 RedisControl 类,然后通过调用类中的方法来访问 Redis 服务器。例如:
```php
$redis = new RedisControl('127.0.0.1', 6379);
$redis->set('name', 'Tom');
echo $redis->get('name');
$redis->delete('name');
```
在上面的示例中,我们先实例化 RedisControl 类并连接到 Redis 服务器,然后通过 set、get 和 delete 方法来设置、获取和删除缓存数据。您可以根据需要添加其他方法来满足您的业务需求。
相关推荐
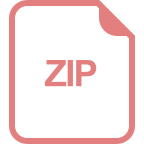












