jsp 保存List的方式
时间: 2023-08-29 21:07:04 浏览: 57
在 JSP 中保存 List 的方式有很多,以下是其中一种常见的方式:
1. 在 JSP 页面中使用 JSTL 标签库进行遍历和输出 List。
```
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
...
<c:forEach var="item" items="${list}">
${item}<br>
</c:forEach>
```
2. 将 List 存储在 ServletRequest、HttpSession 或 ServletContext 中,然后在 JSP 中取出并遍历输出。
在 Servlet 中存储 List:
```
List<String> list = new ArrayList<>();
request.setAttribute("list", list);
```
在 JSP 中取出并遍历输出:
```
<%
List<String> list = (List<String>) request.getAttribute("list");
for (String item : list) {
out.println(item + "<br>");
}
%>
```
3. 使用 AJAX 技术将 List 传递到前端,然后在前端使用 JavaScript 进行遍历和输出。
这些都是比较常见的方式,具体的实现方式可以根据具体场景和需求选择。
相关问题
jsp页面上传图片并保存到数据库中
实现JSP页面上传图片并保存到数据库中的步骤如下:
1. 创建一个带有文件上传表单的JSP页面,例如:
```html
<form action="upload.jsp" method="post" enctype="multipart/form-data">
<input type="file" name="image">
<input type="submit" value="上传">
</form>
```
2. 在上传的JSP页面中,使用Apache Commons FileUpload或Servlet 3.0以上版本提供的API来处理文件上传操作。例如,使用Apache Commons FileUpload可以通过以下方式将上传的文件保存在服务器上:
```java
List<FileItem> items = new ServletFileUpload(new DiskFileItemFactory()).parseRequest(request);
for (FileItem item : items) {
if (!item.isFormField()) {
String fileName = new File(item.getName()).getName();
InputStream fileContent = item.getInputStream();
// 处理文件上传操作
}
}
```
3. 在处理文件上传操作时,将文件内容转换为字节数组,并将字节数组保存到数据库中。例如:
```java
byte[] imageData = IOUtils.toByteArray(fileContent);
// 将字节数组保存到数据库中
```
4. 在保存到数据库之前,需要先连接数据库并打开连接。例如:
```java
Class.forName("com.mysql.jdbc.Driver");
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "password");
```
5. 将字节数组保存到数据库中。这里使用PreparedStatement进行参数化查询,将字节数组作为Blob类型的参数插入到数据库中。例如:
```java
PreparedStatement statement = connection.prepareStatement("INSERT INTO images (name, data) VALUES (?, ?)");
statement.setString(1, fileName);
statement.setBlob(2, new ByteArrayInputStream(imageData));
statement.executeUpdate();
```
6. 处理完文件上传和保存到数据库后,需要关闭连接。例如:
```java
statement.close();
connection.close();
```
以上就是JSP页面上传图片并保存到数据库中的实现步骤。需要注意的是,上传的图片需要进行大小、格式等验证,避免恶意文件上传。同时,需要在web.xml文件中配置multipart-config元素,指定最大文件大小和请求大小限制。
jsp搜索框模糊查询
在JSP页面中实现模糊查询的步骤如下:
1. 在JSP页面中创建一个搜索框,用户可以在搜索框中输入关键字。
2. 当用户点击搜索按钮时,将关键字发送到后台的Servlet。
3. 在Servlet中获取用户输入的关键字。
4. 将关键字进行拼接,添加通配符%,以便进行模糊查询。
5. 调用Dao层的方法,将拼接后的关键字传递给数据库进行查询。
6. 将查询结果保存在一个List集合中。
7. 将List集合通过setAttribute()方法保存在request对象中,以便在JSP页面中使用。
8. 使用EL表达式在JSP页面中获取保存在request对象中的List集合,并依次取出用户信息进行显示。
以下是一个示例代码:
```jsp
<!-- JSP页面的搜索框 -->
<form action="UserServlet" method="GET">
<input type="text" name="keywords" placeholder="请输入关键字">
<input type="submit" value="搜索">
</form>
```
```java
// UserServlet.java
@WebServlet("/UserServlet")
public class UserServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 获取用户输入的关键字
String keywords = request.getParameter("keywords");
// 将关键字进行拼接
String like_keywords = "%" + keywords + "%";
// 调用Dao层的方法,进行模糊查询
List<User> userList = userDao.selectWithLike(like_keywords);
// 将查询结果保存在request对象中
request.setAttribute("userList", userList);
// 请求转发到userlist页面
RequestDispatcher dispatcher = request.getRequestDispatcher("userlist.jsp");
dispatcher.forward(request, response);
}
}
```
```jsp
<!-- userlist.jsp -->
<%-- 使用EL表达式获取保存在request对象中的List集合,并依次取出用户信息进行显示 --%>
<c:forEach items="${userList}" var="user">
<p>${user.name}</p>
<p>${user.age}</p>
<p>${user.email}</p>
</c:forEach>
```
相关推荐
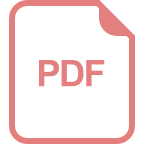
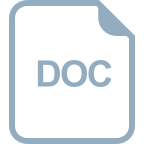












