jsp+javabean实现购物车
时间: 2024-01-26 14:03:32 浏览: 34
以下是使用JSP和JavaBean实现购物车的步骤:
1.创建一个JavaBean类,用于存储商品信息和购物车信息。该类应该包含以下属性:商品ID、商品名称、商品价格、商品数量、购物车总价等。
2.在JSP页面中,使用JSP标签库引入JavaBean类,并创建一个购物车对象。
3.在JSP页面中,使用表单向购物车中添加商品。当用户点击“添加到购物车”按钮时,将商品信息添加到购物车对象中。
4.在JSP页面中,显示购物车中的商品信息和总价。可以使用JSTL标签库来遍历购物车中的商品列表,并计算购物车的总价。
5.在JSP页面中,提供一个“结算”按钮,当用户点击该按钮时,将购物车中的商品信息保存到数据库中,并清空购物车。
以下是一个简单的示例代码:
JavaBean类:
```java
public class Product {
private int id;
private String name;
private double price;
private int quantity;
// 构造函数、getter和setter方法省略
public double getTotalPrice() {
return price * quantity;
}
}
public class ShoppingCart {
private List<Product> productList = new ArrayList<>();
public void addProduct(Product product) {
productList.add(product);
}
public List<Product> getProductList() {
return productList;
}
public double getTotalPrice() {
double totalPrice = 0;
for (Product product : productList) {
totalPrice += product.getTotalPrice();
}
return totalPrice;
}
public void clear() {
productList.clear();
}
}
```
JSP页面:
```jsp
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ page import="com.example.Product,com.example.ShoppingCart" %>
<c:set var="cart" scope="session" value="${sessionScope.cart == null ? new ShoppingCart() : sessionScope.cart}" />
<form action="addProduct.jsp" method="post">
<input type="hidden" name="id" value="1" />
<input type="hidden" name="name" value="Product 1" />
<input type="hidden" name="price" value="10.0" />
<input type="hidden" name="quantity" value="1" />
<input type="submit" value="Add to Cart" />
</form>
<table>
<tr>
<th>ID</th>
<th>Name</th>
<th>Price</th>
<th>Quantity</th>
<th>Total Price</th>
</tr>
<c:forEach items="${cart.productList}" var="product">
<tr>
<td>${product.id}</td>
<td>${product.name}</td>
<td>${product.price}</td>
<td>${product.quantity}</td>
<td>${product.totalPrice}</td>
</tr>
</c:forEach>
<tr>
<td colspan="4">Total Price:</td>
<td>${cart.totalPrice}</td>
</tr>
</table>
<form action="checkout.jsp" method="post">
<input type="submit" value="Checkout" />
</form>
```
addProduct.jsp页面:
```jsp
<%@ page import="com.example.Product,com.example.ShoppingCart" %>
<%
int id = Integer.parseInt(request.getParameter("id"));
String name = request.getParameter("name");
double price = Double.parseDouble(request.getParameter("price"));
int quantity = Integer.parseInt(request.getParameter("quantity"));
Product product = new Product(id, name, price, quantity);
ShoppingCart cart = (ShoppingCart) session.getAttribute("cart");
if (cart == null) {
cart = new ShoppingCart();
session.setAttribute("cart", cart);
}
cart.addProduct(product);
response.sendRedirect("cart.jsp");
%>
```
checkout.jsp页面:
```jsp
<%@ page import="com.example.Product,com.example.ShoppingCart" %>
<%
ShoppingCart cart = (ShoppingCart) session.getAttribute("cart");
// 将购物车中的商品信息保存到数据库中
cart.clear();
response.sendRedirect("cart.jsp");
%>
```
相关推荐
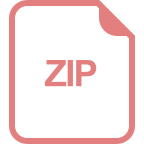
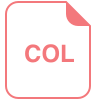













