Javabean+MySQL+servlet清空购物车并刷新页面
时间: 2023-08-15 18:05:05 浏览: 50
实现清空购物车并刷新页面,可以按照以下步骤进行:
1. 在MySQL数据库中创建购物车表,用于存储用户加入购物车的商品信息,表结构如下:
```sql
CREATE TABLE cart (
id INT(11) NOT NULL AUTO_INCREMENT,
user_id INT(11) NOT NULL,
product_id INT(11) NOT NULL,
quantity INT(11) NOT NULL,
PRIMARY KEY (id)
);
```
2. 创建JavaBean类,用于封装购物车中的商品信息。假设该类名为`CartItem`,包含以下属性:
```java
public class CartItem {
private int id;
private int userId;
private int productId;
private int quantity;
// getter和setter方法省略
}
```
3. 创建Servlet,用于处理清空购物车的请求。在Servlet中,首先需要获取当前用户的ID,然后根据用户ID从购物车表中删除所有商品信息,最后将页面重定向到购物车页面。代码如下:
```java
public class ClearCartServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 获取当前用户的ID
int userId = ((User) request.getSession().getAttribute("user")).getId();
// 从购物车表中删除所有商品信息
Connection conn = null;
PreparedStatement ps = null;
try {
conn = DbUtils.getConnection();
String sql = "DELETE FROM cart WHERE user_id=?";
ps = conn.prepareStatement(sql);
ps.setInt(1, userId);
ps.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} finally {
DbUtils.closeQuietly(conn, ps);
}
// 重定向到购物车页面
response.sendRedirect(request.getContextPath() + "/cart.jsp");
}
}
```
4. 在购物车页面中添加清空购物车的按钮,点击该按钮时向Servlet发送清空购物车的请求。代码如下:
```html
<form action="${pageContext.request.contextPath}/clearCart" method="get">
<button type="submit">清空购物车</button>
</form>
```
其中`${pageContext.request.contextPath}`可以获取当前Web应用的根路径。
5. 刷新购物车页面时,需要从购物车表中获取当前用户的所有购物车商品信息,并将其显示在页面上。代码如下:
```java
public class CartServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 获取当前用户的ID
int userId = ((User) request.getSession().getAttribute("user")).getId();
// 从购物车表中获取当前用户的所有购物车商品信息
List<CartItem> cartItems = new ArrayList<>();
Connection conn = null;
PreparedStatement ps = null;
ResultSet rs = null;
try {
conn = DbUtils.getConnection();
String sql = "SELECT * FROM cart WHERE user_id=?";
ps = conn.prepareStatement(sql);
ps.setInt(1, userId);
rs = ps.executeQuery();
while (rs.next()) {
CartItem cartItem = new CartItem();
cartItem.setId(rs.getInt("id"));
cartItem.setUserId(rs.getInt("user_id"));
cartItem.setProductId(rs.getInt("product_id"));
cartItem.setQuantity(rs.getInt("quantity"));
cartItems.add(cartItem);
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
DbUtils.closeQuietly(conn, ps, rs);
}
// 将购物车商品信息传递给页面
request.setAttribute("cartItems", cartItems);
request.getRequestDispatcher("/cart.jsp").forward(request, response);
}
}
```
在页面中,可以通过JSTL标签库来遍历购物车商品信息,并将其显示在页面上。代码如下:
```html
<c:forEach items="${cartItems}" var="cartItem">
<tr>
<td>${cartItem.productId}</td>
<td>${cartItem.quantity}</td>
</tr>
</c:forEach>
```
以上就是使用JavaBean、MySQL和Servlet实现清空购物车并刷新页面的步骤。
相关推荐
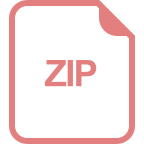
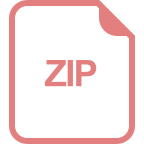
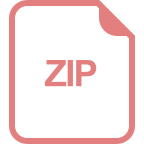














