编写水果管理系统实现增删改查,利用jsp+mysql+javabean+servlet
时间: 2023-09-25 08:11:11 浏览: 55
好的,我可以为你提供一些指导。
首先,你需要创建一个数据库来存储水果信息,包括水果名称、价格、库存等信息。然后,你需要创建一个JavaBean类来表示水果对象,包括属性和方法。接着,你需要编写servlet来处理用户的请求,包括增加、删除、修改、查询水果的请求。最后,你需要利用jsp来展示水果管理系统的界面,包括添加、删除、修改、查询水果的功能。
以下是一个简单的示例:
首先,创建一个名为"fruit"的数据库,并创建一个名为"fruit_info"的表来存储水果信息。表结构如下:
```sql
CREATE TABLE `fruit_info` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(50) NOT NULL,
`price` double NOT NULL,
`stock` int(11) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
```
然后,创建一个JavaBean类"Fruit"来表示水果对象。代码如下:
```java
public class Fruit {
private int id;
private String name;
private double price;
private int stock;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public int getStock() {
return stock;
}
public void setStock(int stock) {
this.stock = stock;
}
}
```
接着,编写一个servlet"FruitServlet"来处理用户的请求。代码如下:
```java
public class FruitServlet extends HttpServlet {
private FruitDao dao = new FruitDao();
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String action = request.getParameter("action");
if ("add".equals(action)) {
addFruit(request, response);
} else if ("delete".equals(action)) {
deleteFruit(request, response);
} else if ("update".equals(action)) {
updateFruit(request, response);
} else if ("search".equals(action)) {
searchFruit(request, response);
} else {
response.sendRedirect("index.jsp");
}
}
private void addFruit(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String name = request.getParameter("name");
double price = Double.parseDouble(request.getParameter("price"));
int stock = Integer.parseInt(request.getParameter("stock"));
Fruit fruit = new Fruit();
fruit.setName(name);
fruit.setPrice(price);
fruit.setStock(stock);
dao.addFruit(fruit);
response.sendRedirect("index.jsp");
}
private void deleteFruit(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int id = Integer.parseInt(request.getParameter("id"));
dao.deleteFruit(id);
response.sendRedirect("index.jsp");
}
private void updateFruit(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int id = Integer.parseInt(request.getParameter("id"));
String name = request.getParameter("name");
double price = Double.parseDouble(request.getParameter("price"));
int stock = Integer.parseInt(request.getParameter("stock"));
Fruit fruit = new Fruit();
fruit.setId(id);
fruit.setName(name);
fruit.setPrice(price);
fruit.setStock(stock);
dao.updateFruit(fruit);
response.sendRedirect("index.jsp");
}
private void searchFruit(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String name = request.getParameter("name");
List<Fruit> fruits = dao.searchFruit(name);
request.setAttribute("fruits", fruits);
request.getRequestDispatcher("search.jsp").forward(request, response);
}
}
```
在servlet中,我们通过判断"action"参数来确定用户的请求,然后调用相应的方法来处理请求。例如,如果用户想要添加水果,我们就调用"addFruit"方法。
在"addFruit"方法中,我们首先获取用户提交的水果信息,然后创建一个Fruit对象,并将水果信息设置到对象中。最后,调用FruitDao中的"addFruit"方法,将水果信息保存到数据库中。
类似的,在其他方法中,我们可以调用FruitDao中的方法来删除、更新、查询水果的信息。
最后,我们需要编写jsp页面来展示水果管理系统的界面。例如,我们可以创建一个名为"index.jsp"的页面来展示水果列表,代码如下:
```html
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<html>
<head>
<title>水果管理系统</title>
</head>
<body>
<h1>水果列表</h1>
<table>
<tr>
<th>ID</th>
<th>名称</th>
<th>价格</th>
<th>库存</th>
<th>操作</th>
</tr>
<c:forEach var="fruit" items="${fruits}">
<tr>
<td>${fruit.id}</td>
<td>${fruit.name}</td>
<td>${fruit.price}</td>
<td>${fruit.stock}</td>
<td>
<a href="update.jsp?id=${fruit.id}&name=${fruit.name}&price=${fruit.price}&stock=${fruit.stock}">修改</a>
<a href="FruitServlet?action=delete&id=${fruit.id}">删除</a>
</td>
</tr>
</c:forEach>
</table>
<h1>添加水果</h1>
<form action="FruitServlet" method="post">
<input type="hidden" name="action" value="add">
<label>名称:</label><input type="text" name="name"><br>
<label>价格:</label><input type="text" name="price"><br>
<label>库存:</label><input type="text" name="stock"><br>
<input type="submit" value="添加">
</form>
<h1>搜索水果</h1>
<form action="FruitServlet" method="post">
<input type="hidden" name="action" value="search">
<label>名称:</label><input type="text" name="name"><br>
<input type="submit" value="搜索">
</form>
</body>
</html>
```
在页面中,我们使用了JSTL标签库来循环展示水果列表。同时,我们也提供了添加、删除、修改、搜索水果的功能。例如,用户可以通过点击"修改"链接来修改水果信息。
以上就是一个简单的水果管理系统的示例。当然,实际开发中还有很多细节需要注意,例如输入参数的验证、异常处理等。
相关推荐
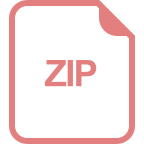
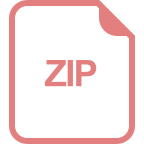
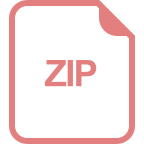
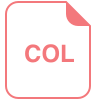













