(Rename files) Suppose you have a lot of files in a directory named Exercisei_j, where i and j are digits. Write a program that pads a 0 before i if i is a single digit. For example, a file named Exercise2_1 in a directory will be renamed to Exercise02_1. In Java, when you pass the symbol * from the command line, it refers to all files in the directory (see Supplement III.V). Use the following command to run your program: java Exercise12_28 *
时间: 2024-03-20 13:38:53 浏览: 66
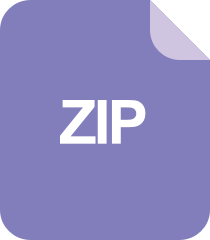
FileReadWrite with text aread output.zip_In Writing_file readers
Sure, I can help you with that! Here's the Java code that should do the job:
```java
import java.io.File;
public class Exercise12_28 {
public static void main(String[] args) {
if (args.length == 0) {
System.out.println("Usage: java Exercise12_28 *");
return;
}
for (String filename : args) {
File file = new File(filename);
String name = file.getName();
String[] parts = name.split("_");
if (parts.length == 2) {
String i = parts[0];
String j = parts[1];
if (i.length() == 1) {
i = "0" + i;
}
String newName = i + "_" + j;
File newFile = new File(file.getParent(), newName);
file.renameTo(newFile);
System.out.println("Renamed " + name + " to " + newName);
}
}
}
}
```
To use it, simply compile the code and run the program with the command `java Exercise12_28 *`. This will pass all the filenames in the current directory as arguments to the program.
The program first checks if any arguments were passed. If not, it displays a usage message and exits. Otherwise, it loops through each filename and performs the following steps:
1. Create a `File` object for the filename.
2. Get the name of the file (without the directory).
3. Split the name into two parts using the underscore as a separator.
4. If the name has two parts, extract the first part as `i` and the second part as `j`.
5. If `i` is a single digit, add a leading zero to it.
6. Construct a new name by combining `i` and `j` with an underscore.
7. Create a new `File` object for the new name in the same directory as the original file.
8. Rename the original file to the new name.
9. Print a message indicating that the file was renamed.
I hope this helps!
阅读全文
相关推荐
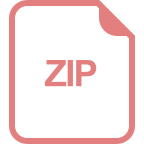
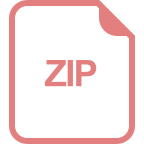


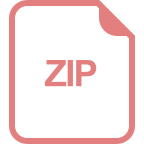
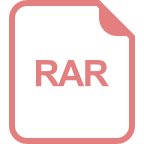
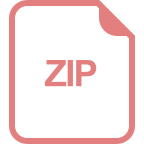
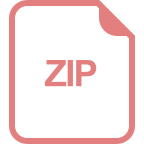
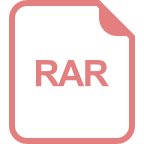
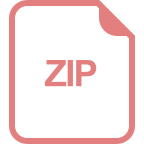
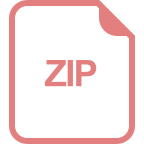
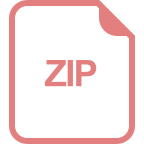
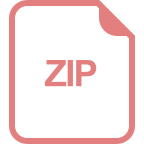
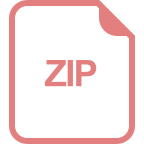
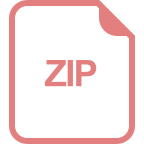