(Replace words) Suppose you have a lot of files in a directory that contain words Exercisei_j, where i and j are digits. Write a program that pads a 0 before i if i is a single digit and 0 before j if j is a single digit. For example, the word Exercise2_1 in a file will be replaced by Exercise02_01. In Java, when you pass the symbol * from the command line, it refers to all files in the directory (see Supplement III.V). Use the following command to run your program: java Exercise12_27 *
时间: 2024-03-08 08:50:15 浏览: 92
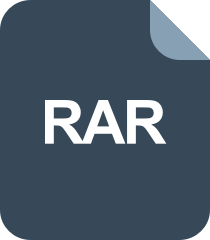
portA_suppose4pm_pic16f877a_
Certainly, I can help you with that. Here's some sample code that should do what you're asking for:
```java
import java.io.File;
public class Exercise12_27 {
public static void main(String[] args) {
if (args.length == 0) {
System.out.println("Please specify a directory or use '*' to apply to all files in the current directory.");
return;
}
String directory = args[0];
File dir = new File(directory);
if (!dir.isDirectory()) {
System.out.println("Invalid directory specified.");
return;
}
File[] files = dir.listFiles();
if (files == null) {
System.out.println("Could not retrieve files in specified directory.");
return;
}
for (File file : files) {
if (file.isFile()) {
String fileName = file.getName();
if (fileName.startsWith("Exercise") && fileName.matches(".*\\d_\\d.*")) {
String[] parts = fileName.split("_");
String i = parts[0].substring(8);
String j = parts[1].substring(0, 1);
if (i.length() == 1) {
i = "0" + i;
}
if (j.length() == 1) {
j = "0" + j;
}
String newFileName = "Exercise" + i + "_" + j + fileName.substring(fileName.indexOf('.'));
File newFile = new File(directory + "/" + newFileName);
if (file.renameTo(newFile)) {
System.out.println("Renamed file " + fileName + " to " + newFileName);
} else {
System.out.println("Could not rename file " + fileName);
}
}
}
}
}
}
```
You can save this code to a file named Exercise12_27.java and then compile and run it using the following commands:
```
javac Exercise12_27.java
java Exercise12_27 *
```
This will execute the program on all files in the current directory that match the pattern Exercisei_j, padding a 0 before i and j if they are single digits. The program will output the names of any files that it successfully renames.
阅读全文
相关推荐
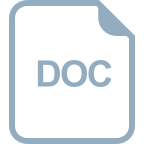
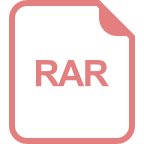
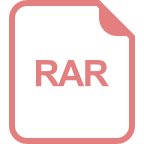
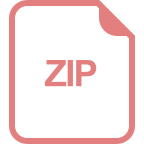
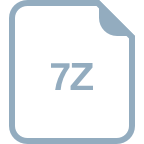
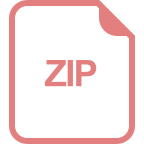
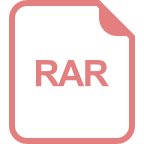
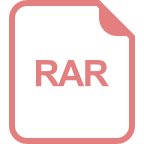
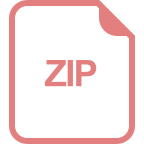