(Split files) Suppose you want to back up a huge file (e.g., a 10-GB AVI file) to a CD-R. You can achieve it by splitting the file into smaller pieces and backing up these pieces separately. Write a utility program that splits a large file into smaller ones using the following command: java Exercise17_10 SourceFile numberOfPieces The command creates the files SourceFile.1, SourceFile.2, . . . , SourceFile.n, where n is numberOfPieces and the output files are about the same size.
时间: 2024-02-23 18:03:16 浏览: 98
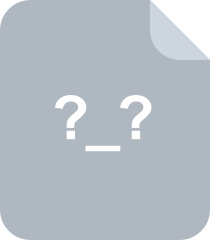
java将大文件分成若干个小文件
Here is the Java code for the utility program that splits a large file into smaller ones:
```java
import java.io.*;
public class Exercise17_10 {
public static void main(String[] args) throws IOException {
if (args.length != 2) {
System.out.println("Usage: java Exercise17_10 SourceFile numberOfPieces");
System.exit(1);
}
String sourceFile = args[0];
int numberOfPieces = Integer.parseInt(args[1]);
File file = new File(sourceFile);
if (!file.exists()) {
System.out.println("Source file " + sourceFile + " does not exist");
System.exit(2);
}
long sourceFileSize = file.length();
long pieceFileSize = sourceFileSize / numberOfPieces + 1;
try (BufferedInputStream input = new BufferedInputStream(new FileInputStream(sourceFile))) {
byte[] buffer = new byte[1024];
for (int i = 1; i <= numberOfPieces; i++) {
String outputFile = sourceFile + "." + i;
try (BufferedOutputStream output = new BufferedOutputStream(new FileOutputStream(outputFile))) {
int count = 0;
while (count < pieceFileSize && input.read(buffer) != -1) {
output.write(buffer);
count += buffer.length;
}
}
}
}
}
}
```
To use the program, run the command `java Exercise17_10 SourceFile numberOfPieces`, where `SourceFile` is the name of the file you want to split, and `numberOfPieces` is the number of pieces you want to split it into. The program will create the files `SourceFile.1`, `SourceFile.2`, ..., `SourceFile.n`, where `n` is `numberOfPieces`. The output files will be about the same size.
阅读全文
相关推荐








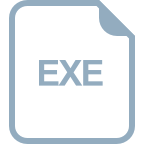
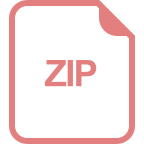
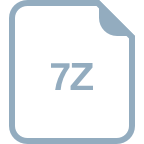
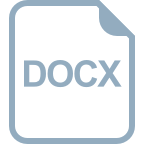