Python Web端向后端发送数据,后端处理后把结果返回web端
时间: 2023-06-28 16:15:29 浏览: 156
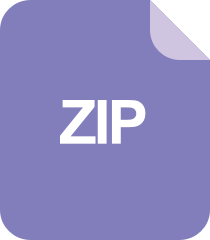
基于Vue框架与Python后端的Web图像增强系统设计源码
好的,你可以使用Python Web框架(如Flask、Django)来实现这个功能。你需要在Web端编写一个前端页面,通过JavaScript将数据发送给后端。后端可以使用Flask或Django的路由功能来接收数据,并根据需要进行处理。最后,后端可以将结果返回给Web端。下面是一个使用Flask框架的示例:
Web端HTML代码:
```
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>发送数据示例</title>
</head>
<body>
<input type="text" id="data">
<button onclick="sendData()">发送</button>
<p id="result"></p>
<script>
function sendData() {
var data = document.getElementById("data").value;
var xhr = new XMLHttpRequest();
xhr.open("POST", "/processData");
xhr.setRequestHeader("Content-Type", "application/json;charset=UTF-8");
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
document.getElementById("result").innerText = xhr.responseText;
}
};
xhr.send(JSON.stringify({"data": data}));
}
</script>
</body>
</html>
```
后端Flask代码:
```
from flask import Flask, request
app = Flask(__name__)
@app.route("/")
def index():
return "Hello, World!"
@app.route("/processData", methods=["POST"])
def processData():
data = request.get_json()["data"]
# 在这里对数据进行处理
result = data.upper()
return result
if __name__ == "__main__":
app.run()
```
这个例子中,Web端页面有一个文本框和一个按钮,用户可以在文本框中输入数据,然后点击按钮发送数据。当用户点击按钮时,JavaScript代码会使用XMLHttpRequest对象向Flask后端的/processData路由发送POST请求,请求体中包含JSON格式的数据。Flask后端接收到请求后,从请求体中获取数据,对数据进行处理,然后将处理结果作为字符串返回给Web端。
阅读全文
相关推荐
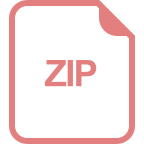
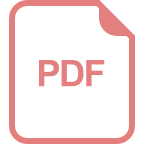
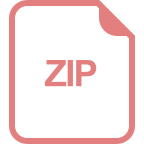
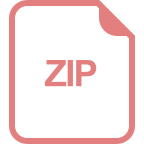
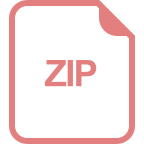
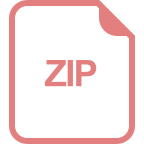
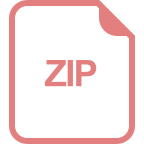
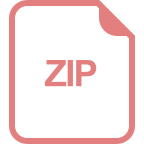
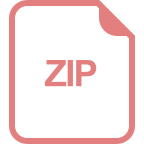
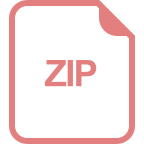
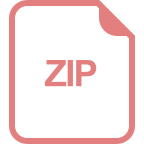
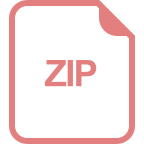
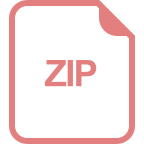
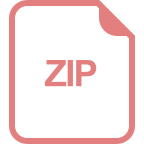
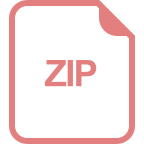
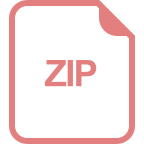
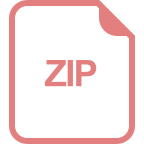
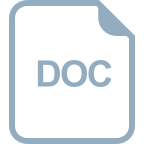