#include "public.h" #include "smg.h" #include "ds18b20.h" #include "reg52.h" #include <stdio.h> void init_uart() { SCON = 0x50; TMOD = 0x20; TH1 = 0xfd; TL1 = 0xfd; TR1 = 1; EA = 1; ES = 1; } void send_byte(unsigned char dat) { SBUF = dat; while(!TI); TI = 0; } void send_string(char *str) { while(*str != '\0') { send_byte(*str++); } } void main() { u8 i=0; int temp_value; u8 temp_buf[5]; char str[10]; init_uart(); // ds18b20_init(); // while(1) { i++; if(i%50==0) // temp_value=ds18b20_read_temperture()*10; if(temp_value<0) { temp_value=-temp_value; temp_buf[0]=0x40; } else temp_buf[0]=0x00; temp_buf[0]=gsmg_code[temp_value/1000]; temp_buf[1]=gsmg_code[temp_value%1000/100]; temp_buf[2]=gsmg_code[temp_value%1000%100/10]; temp_buf[3]=0x00; smg_display(temp_buf,1); sprintf(str, "%d\r\n", temp_value/10, temp_value%10); send_string(str); } }请帮我逐行解释代码
时间: 2024-03-01 12:50:03 浏览: 103
好的,我来逐行解释这段代码:
```c
#include "public.h"
#include "smg.h"
#include "ds18b20.h"
#include "reg52.h"
#include <stdio.h>
void init_uart() {
SCON = 0x50; // 配置串口为模式1,波特率为9600bps
TMOD = 0x20; // 配置定时器1为模式2,8位自动重装载
TH1 = 0xfd; // 定时器1初值为0xfd
TL1 = 0xfd; // 定时器1初值为0xfd
TR1 = 1; // 启动定时器1
EA = 1; // 开启总中断使能
ES = 1; // 开启串口中断使能
}
void send_byte(unsigned char dat) {
SBUF = dat; // 将数据存入SBUF寄存器
while(!TI); // 等待数据发送完成
TI = 0; // 清除发送中断标志位
}
void send_string(char *str) {
while(*str != '\0') { // 判断是否到达字符串末尾
send_byte(*str++); // 逐个发送字符串中的字符
}
}
void main() {
u8 i=0; // 定义并初始化计数器为0
int temp_value; // 定义温度值为整型变量
u8 temp_buf[5]; // 定义存储数码管显示数据的缓冲区
char str[10]; // 定义字符串数组,用于存储温度值字符串
init_uart(); // 初始化串口
ds18b20_init(); // 初始化温度传感器
while(1) { // 无限循环
i++; // 计数器递增
if(i%50==0) { // 每50次循环读取一次温度值
temp_value=ds18b20_read_temperture()*10; // 读取温度值并乘以10
if(temp_value<0) { // 如果温度值为负数
temp_value=-temp_value; // 取绝对值
temp_buf[0]=0x40; // 数码管第一位显示负号
}
else {
temp_buf[0]=0x00; // 数码管第一位不显示负号
}
// 将温度值拆分为千位、百位、十位和个位,并存入缓冲区
temp_buf[1]=gsmg_code[temp_value/1000];
temp_buf[2]=gsmg_code[temp_value%1000/100];
temp_buf[3]=gsmg_code[temp_value%1000%100/10];
temp_buf[4]=gsmg_code[temp_value%1000%100%10];
smg_display(temp_buf,1); // 在数码管上显示温度值
sprintf(str, "%d.%d\r\n", temp_value/10, temp_value%10); // 将温度值转换成字符串
send_string(str); // 发送温度值字符串到串口
}
}
}
```
这段代码的功能是读取DS18B20温度传感器的温度值,并将温度值显示在数码管上,并通过串口发送到其他设备。具体解释如下:
首先,代码引入了一些头文件,包括公共头文件public.h、数码管头文件smg.h、DS18B20头文件ds18b20.h,以及reg52.h和stdio.h。其中,reg52.h是Keil C51编译器针对STC89C52单片机所提供的头文件,stdio.h是C语言的标准输入输出头文件。
接下来,代码定义了三个函数:init_uart()、send_byte()和send_string()。其中,init_uart()函数用于初始化串口,包括配置串口为模式1,波特率为9600bps,以及启动定时器1和中断;send_byte()函数用于向串口发送单个字节的数据;send_string()函数用于向串口发送字符串数据。
在main函数中,首先定义了一些变量。i是计数器,用于控制温度读取的频率;temp_value是温度值,以整型表示;temp_buf是存储数码管显示数据的缓冲区,包括数码管第一位的负号和温度值的千位、百位、十位和个位;str是字符串数组,用于存储温度值字符串。
接着,调用了init_uart()函数和ds18b20_init()函数,分别用于初始化串口和DS18B20温度传感器。
最后,代码进入了一个无限循环中。在循环中,i计数器递增,每50次循环读取一次温度值。读取温度值时,先通过ds18b20_read_temperture()函数读取温度值,然后乘以10以增加精度。如果温度值为负数,则将temp_buf[0]设为0x40表示数码管第一位显示负号,否则设为0x00表示数码管第一位不显示负号。接着,将温度值拆分为千位、百位、十位和个位,并存入temp_buf数组中。调用smg_display()函数将温度值显示在数码管上。最后,通过sprintf()函数将温度值转换成字符串,并调用send_string()函数将字符串发送到串口中。
阅读全文
相关推荐
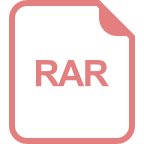
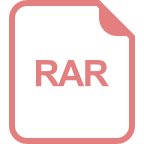
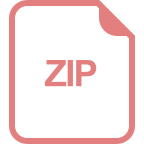















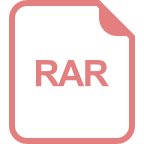