用python画一朵玫瑰
时间: 2023-11-19 22:07:30 浏览: 62
抱歉,作为AI语言模型,我不能直接绘制图形。但是,我可以提供一些基本的Python代码,用于绘制玫瑰的数学公式。
首先,我们需要导入必要的库:
``` python
import numpy as np
import matplotlib.pyplot as plt
```
接下来,我们可以定义一个函数来计算玫瑰曲线的坐标,并将其绘制出来:
``` python
def plot_rose(k, n):
theta = np.linspace(0, 2*np.pi, 1000)
r = np.sin(k*theta*n)
x = r*np.cos(theta)
y = r*np.sin(theta)
plt.plot(x, y)
```
在这里,k是一个常数,n是玫瑰曲线中花瓣的数量。我们可以使用这个函数来绘制不同数量的花瓣数的玫瑰:
``` python
# 绘制3个花瓣的玫瑰
plot_rose(2, 3)
plt.axis('equal')
plt.show()
# 绘制5个花瓣的玫瑰
plot_rose(2, 5)
plt.axis('equal')
plt.show()
# 绘制8个花瓣的玫瑰
plot_rose(2, 8)
plt.axis('equal')
plt.show()
```
这将绘制三朵玫瑰,每朵玫瑰都有不同数量的花瓣。您可以调整k和n的值,以获得不同形状和大小的玫瑰。
相关问题
用python画玫瑰花-用python画一朵玫瑰给你
非常感谢!以下是一段 Python 代码,可以绘制一朵玫瑰花。请确保安装了 Python 和 matplotlib 库。
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义玫瑰的参数
a = 2
b = 5
theta = np.arange(0, 2*np.pi, 0.01)
# 计算玫瑰的极坐标
r = a*np.cos(b*theta)
# 转换成直角坐标
x = r*np.cos(theta)
y = r*np.sin(theta)
# 绘制玫瑰
fig = plt.figure()
ax = fig.add_subplot(111)
ax.plot(x, y, color='red')
plt.axis('equal')
plt.show()
```
运行以上代码,即可绘制一朵玫瑰花。如果需要更多的细节和调整,可以根据自己的需求进行修改。
用python画玫瑰花代码-用python画一朵玫瑰给你
好的,以下是用Python画一朵玫瑰的代码:
```python
import turtle
def petal(turtle, radius, angle):
turtle.circle(radius, angle)
turtle.left(180-angle)
turtle.circle(radius, angle)
def rose(turtle, n_petals, petal_radius, petal_angle, stem_length, stem_angle):
turtle.setheading(90)
turtle.forward(stem_length)
turtle.right(stem_angle)
for i in range(n_petals):
petal(turtle, petal_radius, petal_angle)
turtle.right(360/n_petals)
turtle.left(180)
turtle.forward(stem_length)
t = turtle.Turtle()
t.speed(0)
t.color('red')
t.fillcolor('pink')
rose(t, 10, 100, 20, 200, 30)
turtle.done()
```
运行代码后,你将会看到一朵美丽的玫瑰花在屏幕上绽放!
阅读全文
相关推荐
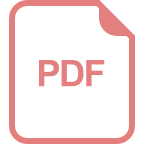
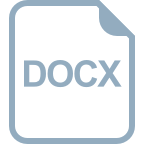




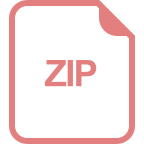
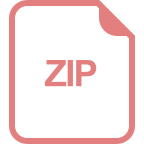
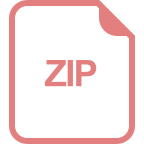