先从键盘录入三行英文文本,然后将其写入文件file1.txt中。I am a studentI am nineteen years oldI am studying at Shandong University of Finance and Economics(提示:将录入的三行英文文本存入一个字符串对象,然后写入文件。)
时间: 2024-03-12 09:48:04 浏览: 94
好的,以下是实现上述功能的Python代码:
```python
# 键盘录入三行英文文本
line1 = input()
line2 = input()
line3 = input()
# 将三行文本拼接为一个字符串
text = line1 + '\n' + line2 + '\n' + line3 + '\n'
# 将字符串写入文件file1.txt中
with open('file1.txt', 'w') as f:
f.write(text)
```
你可以将以上代码复制到Python环境中运行,然后输入三行英文文本,程序会自动将其写入文件file1.txt中。
相关问题
#include<bits/stdc++.h> using namespace std; string str,str1,str2,str3; string ff[9],aa; long long a=0,word[6]={0},out=0; int function(string s,long long &c) { if(s=="one"||s=="a"||s=="first"||s=="another"){word[c]=1;c++;return 0;} if(s=="two"||s=="both"||s=="second"){word[c]=4;c++;return 0;} if(s=="three"||s=="third"){word[c]=9;c++;return 0;} if(s=="four"){word[c]=16;c++;return 0;} if(s=="five"){word[c]=25;c++;return 0;} if(s=="six"){word[c]=36;c++;return 0;} if(s=="seven"){word[c]=49;c++;return 0;} if(s=="eight"){word[c]=64;c++;return 0;} if(s=="nine"){word[c]=81;c++;return 0;} if(s=="eleven"){word[c]=21;c++;return 0;} if(s=="twelve"){word[c]=44;c++;return 0;} if(s=="thirteen"){word[c]=69;c++;return 0;} if(s=="fourteen"){word[c]=96;c++;return 0;} if(s=="fifteen"){word[c]=25;c++;return 0;} if(s=="sixteen"){word[c]=56;c++;return 0;} if(s=="seventeen"){word[c]=89;c++;return 0;} if(s=="eightteen"){word[c]=24;c++;return 0;} if(s=="nineteen"){word[c]=61;c++;return 0;} } int main() { for(int i=0; i<6; i++) { cin>>ff[i]; if (ff[i][ff[i].size()-1]=='.') ff[i].erase(ff[i].size()-1,1); function(ff[i],a); } sort(word,word+a); for(int i=0; i<a; i++) { out+=word[i]; out*=100; } cout<<out/100; return 0; }
这段代码的功能是将输入的字符串中的数字转换为对应的平方数,然后将这些平方数按照从小到大的顺序排列,并输出它们组成的整数。具体实现是通过一个函数来判断每个字符串是否对应一个数字,如果是,则将其转换成平方数,并存储到一个数组中。最后,将数组排序,然后将其中的平方数按顺序组成一个整数输出。
需要注意的是,这段代码可能存在一些问题,比如输入的字符串中可能存在多余的空格或标点符号,可能会影响字符串的判断和转换。此外,该程序中的平方数转换方式也并非完全正确,比如 "fifteen" 对应的平方数应该是 225 而不是 25。
编写窗体应用程序,安排一个文本框、一个按钮和一个标签,从文本框录入一个数字(0~9),点击按钮将其对应的英文单词(如:zero,one等)显示在标签中 。进一步,扩展数据的范围(如:0~100),如何修改程序实现翻译。
以下是使用 C# 编写的窗体应用程序,实现输入数字并将其对应的英文单词显示在标签中:
```csharp
using System;
using System.Windows.Forms;
namespace NumberToWord
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
int num;
if (int.TryParse(textBox1.Text, out num))
{
string word = NumberToWord(num);
label1.Text = word;
}
else
{
MessageBox.Show("请输入有效的数字!");
}
}
private string NumberToWord(int num)
{
string[] ones = { "", "one", "two", "three", "four", "five", "six", "seven", "eight", "nine" };
string[] teens = { "ten", "eleven", "twelve", "thirteen", "fourteen", "fifteen", "sixteen", "seventeen", "eighteen", "nineteen" };
string[] tens = { "", "ten", "twenty", "thirty", "forty", "fifty", "sixty", "seventy", "eighty", "ninety" };
if (num < 0 || num > 100)
{
return "请输入 0 到 100 之间的数字!";
}
else if (num == 0)
{
return "zero";
}
else if (num < 10)
{
return ones[num];
}
else if (num < 20)
{
return teens[num - 10];
}
else if (num < 100)
{
return tens[num / 10] + " " + ones[num % 10];
}
else // num == 100
{
return "one hundred";
}
}
}
}
```
当用户点击按钮时,程序会调用 `NumberToWord` 函数将数字转换成英文单词,并将结果显示在标签中。如果用户输入的数字不在 0 到 100 的范围内,程序会弹出一个提示框。
如果要扩展数据的范围到 0 到 999,可以修改 `NumberToWord` 函数如下:
```csharp
private string NumberToWord(int num)
{
string[] ones = { "", "one", "two", "three", "four", "five", "six", "seven", "eight", "nine" };
string[] teens = { "ten", "eleven", "twelve", "thirteen", "fourteen", "fifteen", "sixteen", "seventeen", "eighteen", "nineteen" };
string[] tens = { "", "ten", "twenty", "thirty", "forty", "fifty", "sixty", "seventy", "eighty", "ninety" };
string[] thousands = { "", "thousand", "million", "billion", "trillion" };
if (num < 0 || num > 999)
{
return "请输入 0 到 999 之间的数字!";
}
else if (num < 10)
{
return ones[num];
}
else if (num < 20)
{
return teens[num - 10];
}
else if (num < 100)
{
return tens[num / 10] + " " + ones[num % 10];
}
else if (num < 1000)
{
return ones[num / 100] + " hundred " + NumberToWord(num % 100);
}
else // num == 1000 or larger
{
for (int i = thousands.Length - 1; i >= 1; i--)
{
int divisor = (int)Math.Pow(1000, i);
if (num >= divisor)
{
return NumberToWord(num / divisor) + " " + thousands[i] + " " + NumberToWord(num % divisor);
}
}
return "one thousand";
}
}
```
在这个新的实现中,我们使用了 `thousands` 数组来存储千位及以上的单位,然后使用递归的方式将数字分解成千位及以下的部分,并将它们转换成英文单词。注意,在处理千位及以上的部分时,我们使用了循环来遍历 `thousands` 数组,并找到最大的千位及以上的单位。
阅读全文
相关推荐
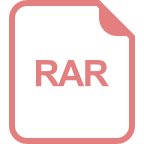
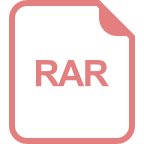
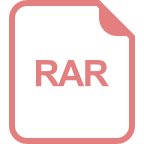
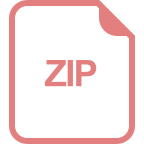
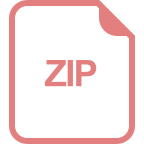
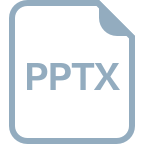
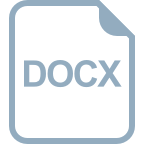
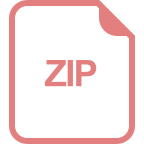
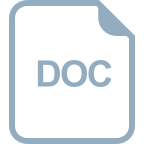
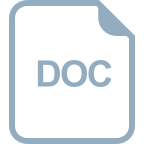

