使用C语言根据后序遍历和中序遍历创建二叉树中序遍历数组名为in后序遍历数组名为post数组长度为n根节点为T
时间: 2024-04-30 21:22:01 浏览: 121
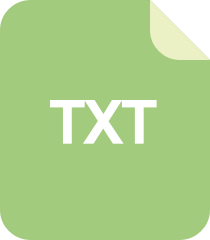
二叉树遍历输出
以下是使用C语言根据后序遍历和中序遍历创建二叉树的代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct TreeNode {
int val;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
TreeNode* buildTree(int* inorder, int inStart, int inEnd, int* postorder, int postStart, int postEnd)
{
if (inStart > inEnd || postStart > postEnd) {
return NULL;
}
int rootVal = postorder[postEnd];
int rootIndex = -1;
for (int i = inStart; i <= inEnd; i++) {
if (inorder[i] == rootVal) {
rootIndex = i;
break;
}
}
TreeNode* root = (TreeNode*)malloc(sizeof(TreeNode));
root->val = rootVal;
root->left = buildTree(inorder, inStart, rootIndex - 1, postorder, postStart, postStart + rootIndex - inStart - 1);
root->right = buildTree(inorder, rootIndex + 1, inEnd, postorder, postStart + rootIndex - inStart, postEnd - 1);
return root;
}
int main()
{
int in[] = { 4, 2, 5, 1, 6, 3 };
int post[] = { 4, 5, 2, 6, 3, 1 };
int n = sizeof(in) / sizeof(in[0]);
TreeNode* root = buildTree(in, 0, n - 1, post, 0, n - 1);
return 0;
}
```
代码中的 `buildTree` 函数接收中序遍历数组 `in`、后序遍历数组 `post` 的起始和结束下标,返回根节点。函数首先判断边界条件,当 `inStart > inEnd` 或 `postStart > postEnd` 时,返回 NULL。接着,在后序遍历数组中找到当前子树的根节点值 `rootVal`,并在中序遍历数组中找到 `rootVal` 对应的下标 `rootIndex`。根据 `rootIndex`,可以将中序遍历数组分成左子树和右子树两部分。同时,由于后序遍历的顺序是左子树、右子树、根节点,所以在后序遍历数组中也可以分出左子树和右子树。接着,递归调用 `buildTree` 函数,分别构建左子树和右子树,并将其作为当前根节点的左右子节点。最后,返回根节点。
在 `main` 函数中,我们可以定义中序遍历数组 `in` 和后序遍历数组 `post`,并将其传入 `buildTree` 函数。代码执行完毕后,我们就得到了一棵二叉树的根节点。
阅读全文
相关推荐
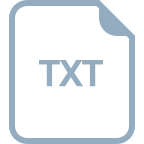
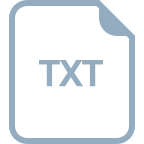















