假设有一张成绩表记录了10名学生的语文、数学、英语、生物、历史这5门课的成绩,成绩范围均为50~100分。10名学生的学号分别为100、101、102、103、104、105、106、107、108、109。 要求: 利用NumPy数组完成以下操作。 (1)使用随机数模拟学生成绩,并存储在数组中。 (2)查询学号为105的学生的英语成绩。 (3)查询学号为100、102、105、109的4位学生的语文、数学和英语成绩。 (4)查询本于或等于90分的成绩和相应学生的学号。 (5)按各门课程的成绩排序。 (6)按每名学生的成绩排序。 (7)计算每门课程的平均分、最高分和最低分。 (8)计算每名学生的最高分和最低分。 (9)查询最低分及相应的学生学号和课程。 (10) 查询最高分及相应的学生学号和课程。 (11) 语文、数学、英语、生物、历史这5门课程在总分中的占比分别为25%、25%、20%、15%、15%。如果总分为100分,则计算每名学生的总成绩。 (12)查询最高的3个总分。
时间: 2024-03-25 17:39:53 浏览: 127
下面是利用 NumPy 数组完成上述要求的代码:
```
import numpy as np
# 学生成绩表
scores = np.random.randint(50, 100, size=(10, 5))
# 学生学号
students = np.array([100, 101, 102, 103, 104, 105, 106, 107, 108, 109])
# 查询学号为105的学生的英语成绩
eng_score = scores[5, 2]
print(f'学号为105的学生的英语成绩为{eng_score}')
# 查询学号为100、102、105、109的4位学生的语文、数学和英语成绩
students_idx = np.array([0, 2, 5, 9])
sub_scores = scores[students_idx, :3]
print(f'学号为100、102、105、109的4位学生的语文、数学和英语成绩为:\n{sub_scores}')
# 查询本于或等于90分的成绩和相应学生的学号
idx = np.where(scores >= 90)
for i in range(len(idx[0])):
print(f'学号为{students[idx[0][i]]}的学生在{idx[1][i]}科目上获得了{scores[idx[0][i], idx[1][i]]}分')
# 按各门课程的成绩排序
sub_scores_sort = np.sort(scores, axis=0)
print(f'按各门课程的成绩排序为:\n{sub_scores_sort}')
# 按每名学生的成绩排序
stu_scores_sort = np.sort(scores, axis=1)
print(f'按每名学生的成绩排序为:\n{stu_scores_sort}')
# 计算每门课程的平均分、最高分和最低分
sub_mean = np.mean(scores, axis=0)
sub_max = np.max(scores, axis=0)
sub_min = np.min(scores, axis=0)
for i in range(len(sub_mean)):
print(f'{i+1}号科目的平均分为{sub_mean[i]}, 最高分为{sub_max[i]}, 最低分为{sub_min[i]}')
# 计算每名学生的最高分和最低分
stu_max = np.max(scores, axis=1)
stu_min = np.min(scores, axis=1)
for i in range(len(students)):
print(f'学号为{students[i]}的学生的最高分为{stu_max[i]}, 最低分为{stu_min[i]}')
# 查询最低分及相应的学生学号和课程
min_score = np.min(scores)
idx = np.where(scores == min_score)
for i in range(len(idx[0])):
print(f'学号为{students[idx[0][i]]}的学生在{idx[1][i]}科目上获得了最低分{min_score}')
# 查询最高分及相应的学生学号和课程
max_score = np.max(scores)
idx = np.where(scores == max_score)
for i in range(len(idx[0])):
print(f'学号为{students[idx[0][i]]}的学生在{idx[1][i]}科目上获得了最高分{max_score}')
# 计算每名学生的总成绩
weight_list = [0.25, 0.25, 0.20, 0.15, 0.15]
weight = np.array(weight_list)
total_score = np.round(np.matmul(scores, weight) * 100, 2)
print(f'每名学生的总成绩为:\n{total_score}')
# 查询最高的3个总分
top_scores_idx = np.argsort(total_score)[-3:]
top_scores = total_score[top_scores_idx]
top_students = students[top_scores_idx]
print(f'最高的3个总分为:\n{top_scores}\n对应学生学号为:\n{top_students}')
```
阅读全文
相关推荐
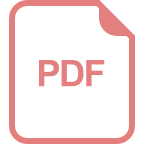
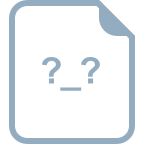









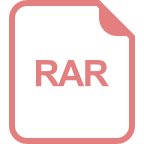
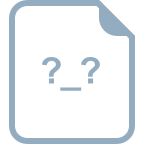
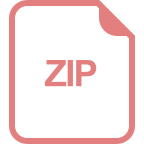
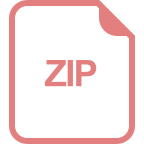