哈希表的增删改查C语言
时间: 2023-07-04 19:19:38 浏览: 163
哈希表是一种常用的数据结构,可以用于快速查找和插入数据。以下是哈希表的增删改查的 C 语言实现:
1. 哈希表的初始化
```c
#define TABLE_SIZE 1000 // 哈希表的大小
typedef struct HashNode {
char* key;
int value;
struct HashNode* next;
} HashNode;
typedef struct HashTable {
HashNode** table;
} HashTable;
HashTable* createHashTable() {
HashTable* ht = (HashTable*)malloc(sizeof(HashTable));
ht->table = (HashNode**)calloc(TABLE_SIZE, sizeof(HashNode*));
return ht;
}
```
2. 哈希函数
哈希函数将字符串转换为哈希表中的索引值。一种简单的哈希函数是将每个字符的 ASCII 码值相加,并取余哈希表的大小。
```c
int hash(char* key) {
int sum = 0;
while (*key != '\0') {
sum += *key;
key++;
}
return sum % TABLE_SIZE;
}
```
3. 插入元素
插入元素时,先计算哈希值,然后在哈希表中查找对应的链表。如果链表中已经存在相同的键,更新值即可;如果不存在相同的键,则在链表头插入新的节点。
```c
void put(HashTable* ht, char* key, int value) {
int index = hash(key);
HashNode* node = ht->table[index];
while (node != NULL) {
if (strcmp(node->key, key) == 0) {
node->value = value;
return;
}
node = node->next;
}
HashNode* new_node = (HashNode*)malloc(sizeof(HashNode));
new_node->key = key;
new_node->value = value;
new_node->next = ht->table[index];
ht->table[index] = new_node;
}
```
4. 查找元素
查找元素时,先计算哈希值,然后在哈希表中查找对应的链表。如果链表中存在相同的键,返回对应的值;如果不存在相同的键,返回 NULL。
```c
int* get(HashTable* ht, char* key) {
int index = hash(key);
HashNode* node = ht->table[index];
while (node != NULL) {
if (strcmp(node->key, key) == 0) {
return &node->value;
}
node = node->next;
}
return NULL;
}
```
5. 删除元素
删除元素时,先计算哈希值,然后在哈希表中查找对应的链表。如果链表中存在相同的键,删除对应的节点;如果不存在相同的键,什么也不做。
```c
void remove(HashTable* ht, char* key) {
int index = hash(key);
HashNode* node = ht->table[index];
HashNode* prev = NULL;
while (node != NULL) {
if (strcmp(node->key, key) == 0) {
if (prev == NULL) {
ht->table[index] = node->next;
} else {
prev->next = node->next;
}
free(node);
return;
}
prev = node;
node = node->next;
}
}
```
阅读全文
相关推荐

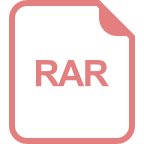
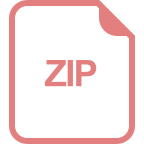
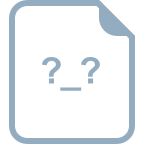
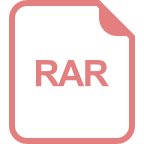
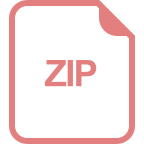
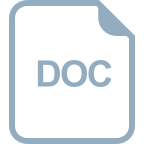
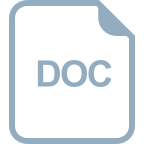



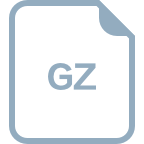
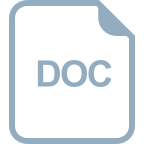
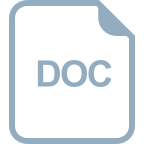

