用c语言代码做班级通讯查询系统,基本要求,数据为本班全体同学信息,存储方式采用哈希表,可增、删、改信息,并可按电话号码和姓名查找,然后要可以文件读写
时间: 2024-03-16 22:47:38 浏览: 98
好的,下面是一个简单的班级通讯查询系统的C语言代码,基于哈希表实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100 // 哈希表的最大容量
typedef struct Student {
char name[20];
char phone[20];
} Student;
typedef struct HashTable {
Student *students[MAX_SIZE];
int count;
} HashTable;
int hash(char *str) {
int sum = 0;
for (int i = 0; i < strlen(str); i++) {
sum += str[i];
}
return sum % MAX_SIZE;
}
void insert(HashTable *ht, Student *s) {
int index = hash(s->phone);
while (ht->students[index] != NULL) {
index = (index + 1) % MAX_SIZE;
}
ht->students[index] = s;
ht->count++;
}
Student *search(HashTable *ht, char *key) {
int index = hash(key);
while (ht->students[index] != NULL) {
if (strcmp(ht->students[index]->phone, key) == 0 || strcmp(ht->students[index]->name, key) == 0) {
return ht->students[index];
}
index = (index + 1) % MAX_SIZE;
}
return NULL;
}
void delete(HashTable *ht, char *key) {
int index = hash(key);
while (ht->students[index] != NULL) {
if (strcmp(ht->students[index]->phone, key) == 0 || strcmp(ht->students[index]->name, key) == 0) {
ht->students[index] = NULL;
ht->count--;
return;
}
index = (index + 1) % MAX_SIZE;
}
}
void update(HashTable *ht, char *key, char *new_name, char *new_phone) {
Student *s = search(ht, key);
if (s != NULL) {
strcpy(s->name, new_name);
strcpy(s->phone, new_phone);
}
}
void save(HashTable *ht) {
FILE *fp = fopen("students.txt", "w");
if (fp == NULL) {
printf("Error: cannot open file\n");
return;
}
for (int i = 0; i < MAX_SIZE; i++) {
if (ht->students[i] != NULL) {
fprintf(fp, "%s %s\n", ht->students[i]->name, ht->students[i]->phone);
}
}
fclose(fp);
}
void load(HashTable *ht) {
FILE *fp = fopen("students.txt", "r");
if (fp == NULL) {
printf("Error: cannot open file\n");
return;
}
char name[20], phone[20];
while (fscanf(fp, "%s %s", name, phone) != EOF) {
Student *s = (Student *)malloc(sizeof(Student));
strcpy(s->name, name);
strcpy(s->phone, phone);
insert(ht, s);
}
fclose(fp);
}
void print_menu() {
printf("1. Add student\n");
printf("2. Search student\n");
printf("3. Delete student\n");
printf("4. Update student\n");
printf("5. Save to file\n");
printf("6. Load from file\n");
printf("7. Exit\n");
}
int main() {
HashTable ht = {0};
int choice;
char name[20], phone[20];
while (1) {
print_menu();
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("Enter student name: ");
scanf("%s", name);
printf("Enter student phone: ");
scanf("%s", phone);
Student *s = (Student *)malloc(sizeof(Student));
strcpy(s->name, name);
strcpy(s->phone, phone);
insert(&ht, s);
break;
case 2:
printf("Enter search key: ");
scanf("%s", phone);
Student *result = search(&ht, phone);
if (result != NULL) {
printf("Name: %s\nPhone: %s\n", result->name, result->phone);
} else {
printf("Not found\n");
}
break;
case 3:
printf("Enter delete key: ");
scanf("%s", phone);
delete(&ht, phone);
break;
case 4:
printf("Enter search key: ");
scanf("%s", phone);
result = search(&ht, phone);
if (result != NULL) {
printf("Enter new name: ");
scanf("%s", name);
printf("Enter new phone: ");
scanf("%s", phone);
update(&ht, result->phone, name, phone);
} else {
printf("Not found\n");
}
break;
case 5:
save(&ht);
break;
case 6:
load(&ht);
break;
case 7:
exit(0);
default:
printf("Invalid choice\n");
break;
}
}
return 0;
}
```
这个代码实现了基本的增删改查功能,同时支持保存到文件和从文件读取。你可以根据自己的需要进行修改和扩展。
阅读全文
相关推荐
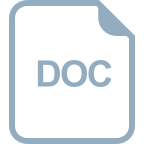
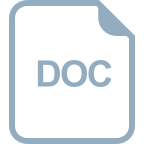
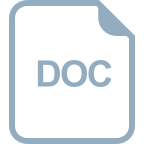

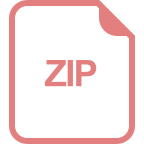
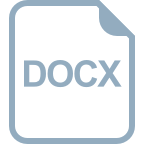
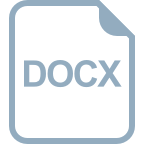
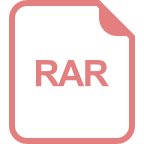
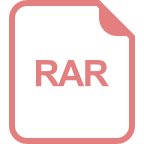
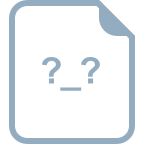
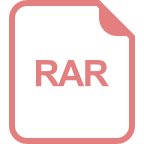
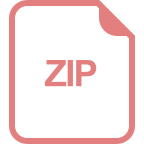
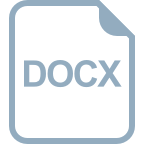
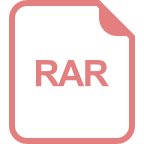
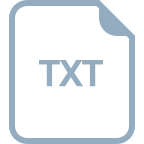
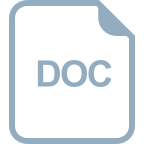