用c语言写一个用哈希表的电话号码查询系统
时间: 2024-03-14 11:47:09 浏览: 99
好的,下面是一个简单的用哈希表实现的电话号码查询系统的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NAME_LEN 20
#define MAX_PHONE_LEN 11
// 定义哈希表结构体
typedef struct _hash_table {
char name[MAX_NAME_LEN];
char phone[MAX_PHONE_LEN];
struct _hash_table *next;
} hash_table;
// 计算哈希值的函数
int hash(char *str) {
int hash_val = 0;
for (int i = 0; i < strlen(str); i++) {
hash_val += str[i];
}
return hash_val % 100; // 哈希表大小为100
}
// 添加联系人到哈希表
void add_contact(hash_table **table, char *name, char *phone) {
int index = hash(name);
// 创建新节点
hash_table *new_node = malloc(sizeof(hash_table));
strcpy(new_node->name, name);
strcpy(new_node->phone, phone);
new_node->next = NULL;
// 如果该位置没有节点,则直接插入
if (table[index] == NULL) {
table[index] = new_node;
} else {
// 如果该位置已经有节点,则插入到链表尾部
hash_table *cur_node = table[index];
while (cur_node->next != NULL) {
cur_node = cur_node->next;
}
cur_node->next = new_node;
}
}
// 从哈希表中查找联系人
char *find_contact(hash_table **table, char *name) {
int index = hash(name);
hash_table *cur_node = table[index];
// 遍历链表查找联系人
while (cur_node != NULL) {
if (strcmp(cur_node->name, name) == 0) {
return cur_node->phone;
}
cur_node = cur_node->next;
}
return "Not found.";
}
int main() {
// 初始化哈希表
hash_table **table = malloc(sizeof(hash_table*) * 100);
for (int i = 0; i < 100; i++) {
table[i] = NULL;
}
// 添加联系人
add_contact(table, "张三", "12345678901");
add_contact(table, "李四", "23456789012");
add_contact(table, "王五", "34567890123");
// 查找联系人
printf("%s\n", find_contact(table, "张三"));
printf("%s\n", find_contact(table, "李四"));
printf("%s\n", find_contact(table, "王五"));
printf("%s\n", find_contact(table, "赵六"));
// 释放内存
for (int i = 0; i < 100; i++) {
hash_table *cur_node = table[i];
while (cur_node != NULL) {
hash_table *next_node = cur_node->next;
free(cur_node);
cur_node = next_node;
}
}
free(table);
return 0;
}
```
上述代码中,我们使用哈希表来存储联系人的姓名和电话号码,哈希表的大小为100。我们首先定义了一个哈希表结构体,包含了姓名、电话号码和下一个节点的指针。然后我们定义了一个计算哈希值的函数,使用字符串的ASCII码之和对哈希表大小取模来得到哈希值。
接着,我们实现了添加联系人和查找联系人的函数。添加联系人时,我们首先计算其哈希值,然后创建一个新节点,并将其插入到哈希表对应位置的链表尾部。查找联系人时,我们根据姓名计算其哈希值,然后遍历哈希表对应位置的链表,查找是否有该联系人。
最后,我们在main函数中添加了几个联系人,并对其进行查找。注意,在程序结束前,我们需要释放哈希表中所有节点的内存。
阅读全文
相关推荐
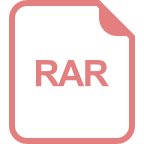
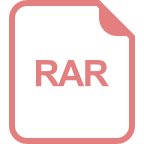

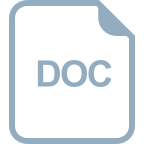

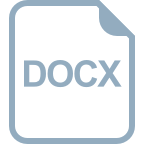
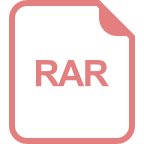
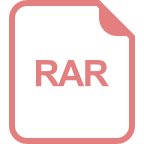
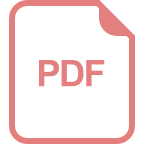
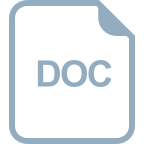
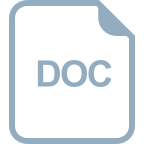





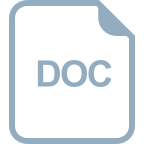
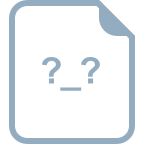