班级通讯查询系统,基本要求,数据为本班全体同学信息,存储方式采用哈希表,可增、删、改信息,并可按电话号码和姓名查找
时间: 2024-03-08 16:45:11 浏览: 58
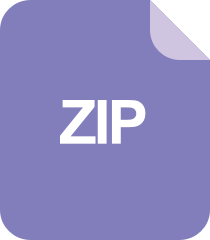
可以在文件里面存储学生信息,可以建立并删除学生成绩,可以按班级查询学生成绩.zip
好的,针对您的问题,我可以给出以下建议:
1. 数据结构:存储方式采用哈希表,可以使用C++ STL中的unordered_map来实现。其中,键值为学生的电话号码或姓名,值为学生的信息,可以定义一个结构体来存储学生信息。
2. 增加学生信息:可以通过键值对的方式将学生信息插入哈希表中,即使用insert()函数。在插入前,需要判断该学生信息是否已经存在,可以使用find()函数进行查找。
3. 删除学生信息:同样可以使用erase()函数来删除学生信息,需要指定要删除的键值。
4. 修改学生信息:可以通过查找到要修改的学生信息,然后进行修改,再将修改后的信息插入到哈希表中的方式来实现。
5. 按电话号码和姓名查找:可以使用find()函数来查找对应的学生信息,需要指定要查找的键值。如果需要按照姓名查找,则需要先将学生信息按照姓名排序,可以使用STL中的sort()函数来实现。
以上是建议,您可以根据实际情况进行调整和完善。
阅读全文
相关推荐
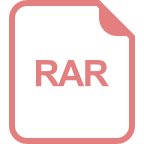
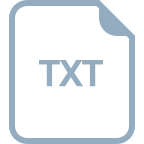
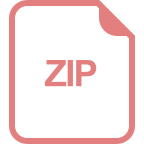
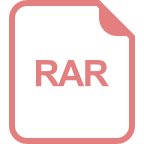
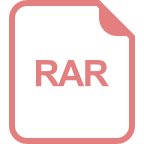
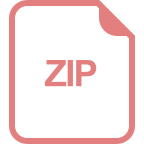
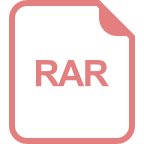
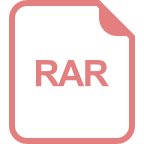
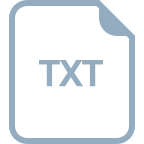
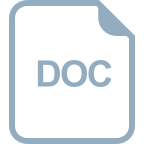
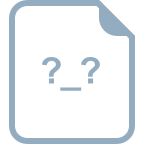
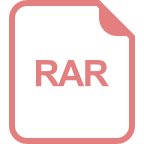