java复制本机文件夹中的所有文件和文件夹到另一个服务器中的指定文件夹中
时间: 2023-05-12 09:03:52 浏览: 58
你可以使用 Java 的 File 类和 IO 流来实现复制本机文件夹中的所有文件和文件夹到另一个服务器中的指定文件夹中。具体实现可以参考以下代码:
```
import java.io.*;
public class FileCopy {
public static void main(String[] args) throws IOException {
String srcPath = "C:/srcFolder"; // 源文件夹路径
String destPath = "D:/destFolder"; // 目标文件夹路径
File srcFolder = new File(srcPath);
File destFolder = new File(destPath);
// 如果目标文件夹不存在,则创建
if (!destFolder.exists()) {
destFolder.mkdir();
}
// 获取源文件夹下的所有文件和文件夹
File[] files = srcFolder.listFiles();
// 遍历源文件夹下的所有文件和文件夹
for (File file : files) {
if (file.isFile()) { // 如果是文件,则复制文件
FileInputStream fis = new FileInputStream(file);
FileOutputStream fos = new FileOutputStream(destPath + "/" + file.getName());
byte[] buffer = new byte[1024];
int len;
while ((len = fis.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
fis.close();
fos.close();
} else if (file.isDirectory()) { // 如果是文件夹,则递归复制文件夹
String subSrcPath = srcPath + "/" + file.getName();
String subDestPath = destPath + "/" + file.getName();
File subSrcFolder = new File(subSrcPath);
File subDestFolder = new File(subDestPath);
copyFolder(subSrcFolder, subDestFolder);
}
}
}
// 递归复制文件夹
public static void copyFolder(File srcFolder, File destFolder) throws IOException {
if (srcFolder.isDirectory()) {
if (!destFolder.exists()) {
destFolder.mkdir();
}
File[] files = srcFolder.listFiles();
for (File file : files) {
if (file.isFile()) {
FileInputStream fis = new FileInputStream(file);
FileOutputStream fos = new FileOutputStream(destFolder.getPath() + "/" + file.getName());
byte[] buffer = new byte[1024];
int len;
while ((len = fis.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
fis.close();
fos.close();
} else if (file.isDirectory()) {
String subSrcPath = srcFolder.getPath() + "/" + file.getName();
String subDestPath = destFolder.getPath() + "/" + file.getName();
File subSrcFolder = new File(subSrcPath);
File subDestFolder = new File(subDestPath);
copyFolder(subSrcFolder, subDestFolder);
}
}
}
}
}
```
这段代码可以将本机的 `C:/srcFolder` 文件夹中的所有文件和文件夹复制到另一个服务器的 `D:/destFolder` 文件夹中。
相关推荐
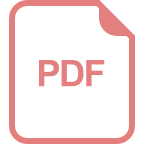
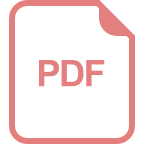














