数据结构实验6:C++实现二叉树类
时间: 2023-07-07 22:25:13 浏览: 124
好的,我可以回答你关于数据结构实验6的问题。C++实现二叉树类的具体实现如下:
```cpp
#include <iostream>
using namespace std;
// 节点结构体
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(nullptr), right(nullptr) {}
};
// 二叉树类
class BinaryTree {
public:
BinaryTree() : root(nullptr) {}
// 插入节点
void insert(int val) {
if (root == nullptr) {
root = new TreeNode(val);
return;
}
TreeNode* cur = root;
while (cur != nullptr) {
if (val < cur->val) {
if (cur->left == nullptr) {
cur->left = new TreeNode(val);
return;
}
cur = cur->left;
} else {
if (cur->right == nullptr) {
cur->right = new TreeNode(val);
return;
}
cur = cur->right;
}
}
}
// 查找节点
bool find(int val) {
TreeNode* cur = root;
while (cur != nullptr) {
if (cur->val == val) {
return true;
} else if (cur->val > val) {
cur = cur->left;
} else {
cur = cur->right;
}
}
return false;
}
// 删除节点
void remove(int val) {
root = removeNode(root, val);
}
// 中序遍历
void inorder() {
inorderTraversal(root);
}
private:
// 根节点
TreeNode* root;
// 删除节点
TreeNode* removeNode(TreeNode* root, int val) {
if (root == nullptr) return root;
if (val < root->val) {
root->left = removeNode(root->left, val);
} else if (val > root->val) {
root->right = removeNode(root->right, val);
} else {
if (root->left == nullptr) {
TreeNode* rightChild = root->right;
delete root;
return rightChild;
} else if (root->right == nullptr) {
TreeNode* leftChild = root->left;
delete root;
return leftChild;
} else {
TreeNode* minNode = findMin(root->right);
root->val = minNode->val;
root->right = removeNode(root->right, minNode->val);
}
}
return root;
}
// 查找最小节点
TreeNode* findMin(TreeNode* root) {
while (root->left != nullptr) {
root = root->left;
}
return root;
}
// 中序遍历
void inorderTraversal(TreeNode* root) {
if (root == nullptr) return;
inorderTraversal(root->left);
cout << root->val << " ";
inorderTraversal(root->right);
}
};
```
以上是基本的二叉树类实现,包括了插入节点、查找节点、删除节点和中序遍历等操作。你可以根据需要进行修改和扩展。
阅读全文
相关推荐
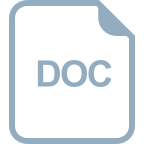
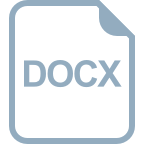
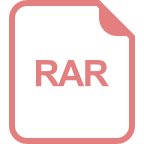
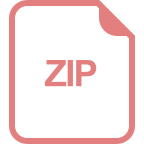
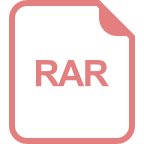
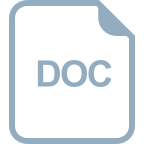
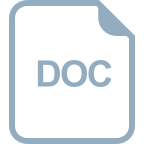
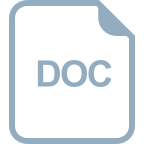
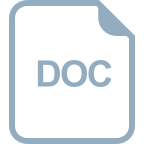
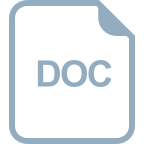
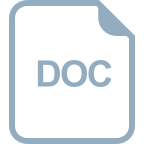
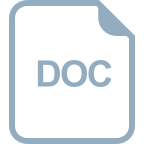
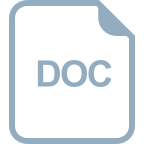
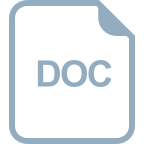
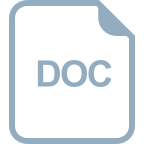
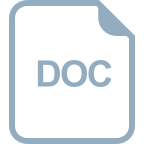