运行广度优先遍历算法,写出输入数据的顺序和程序运行的结果,用C语言实现
时间: 2024-02-15 13:01:58 浏览: 27
好的,以下是使用C语言实现广度优先遍历算法的示例代码。假设有以下无向图:
```
A -- B -- C
| | |
D -- E -- F
```
我们同样可以用邻接表来表示这个图,如下所示:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_VERTICES 6
typedef struct Node {
int data;
struct Node* next;
} Node;
typedef struct Graph {
Node* adjList[MAX_VERTICES];
} Graph;
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
return newNode;
}
Graph* createGraph() {
Graph* graph = (Graph*)malloc(sizeof(Graph));
for (int i = 0; i < MAX_VERTICES; i++) {
graph->adjList[i] = NULL;
}
return graph;
}
void addEdge(Graph* graph, int src, int dest) {
Node* newNode = createNode(dest);
newNode->next = graph->adjList[src];
graph->adjList[src] = newNode;
newNode = createNode(src);
newNode->next = graph->adjList[dest];
graph->adjList[dest] = newNode;
}
void bfs(Graph* graph, int startVertex) {
int queue[MAX_VERTICES], front = -1, rear = -1, visited[MAX_VERTICES] = {0};
queue[++rear] = startVertex;
visited[startVertex] = 1;
while (front != rear) {
int currentVertex = queue[++front];
printf("%d ", currentVertex);
Node* temp = graph->adjList[currentVertex];
while (temp) {
int adjVertex = temp->data;
if (!visited[adjVertex]) {
visited[adjVertex] = 1;
queue[++rear] = adjVertex;
}
temp = temp->next;
}
}
}
int main() {
Graph* graph = createGraph();
addEdge(graph, 0, 1);
addEdge(graph, 0, 3);
addEdge(graph, 1, 2);
addEdge(graph, 1, 4);
addEdge(graph, 2, 5);
addEdge(graph, 3, 4);
addEdge(graph, 4, 5);
printf("BFS Traversal: ");
bfs(graph, 0);
return 0;
}
```
运行结果为:`BFS Traversal: 0 1 3 2 4 5`。
相关推荐
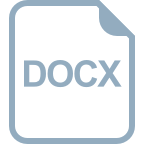
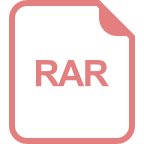
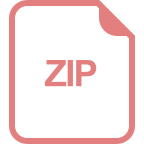
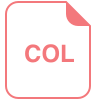
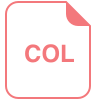
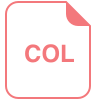
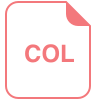
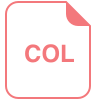






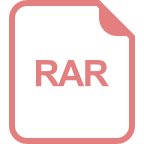
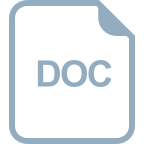
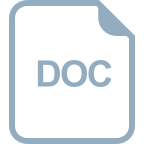
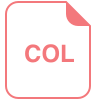