v.pics.forEach((u, i) => { if (u.split('/')[0] == 'cloud:') { } else { picCursor.push(i); wx.getFileSystemManager().getFileInfo({ filePath: u, success: function (res) { if (res.size > 200 * 1024) { wx.compressImage({ src: u, quality: 30, }).then(res => { const tmpFilePath = res.tempFilePath; tmpPromise.push(wx.cloud.uploadFile({ cloudPath: 'usersproducts/' + upID + Date.now() + (i + '.png'), filePath: tmpFilePath, })); }) } else { tmpPromise.push(wx.cloud.uploadFile({ cloudPath: 'usersproducts/' + upID + Date.now() + (i + '.png'), filePath: u, })) } } }) } });请分析指出这段代码存在哪些问题?
时间: 2024-02-05 09:03:27 浏览: 70
这段代码存在以下问题:
1. 在压缩图片后,没有进行错误处理,如果压缩失败,可能会导致上传失败,因此需要加上 catch 方法来处理异常情况。
2. 在压缩图片后,没有进行清理,可能会导致临时文件占用过多空间,因此需要在上传完成后,手动删除临时文件。
3. 在循环中,每次调用 wx.getFileSystemManager().getFileInfo() 方法都会进行一次文件系统的操作,这会降低程序的性能。因此,可以在循环外部先将需要获取文件信息的文件路径保存下来,然后再进行循环,这样就可以避免重复操作文件系统,提高程序的性能。
4. 在上传文件时,没有进行错误处理,如果上传失败,可能会导致程序出错。因此,需要加上 catch 方法来处理上传文件失败的情况。
综上所述,应该修改为以下代码:
```
// 保存需要获取文件信息的文件路径
const filePaths = v.pics.filter(u => u.split('/')[0] != 'cloud:');
// 获取文件信息
wx.getFileSystemManager().getFileInfo({
filePath: filePaths,
success: function (res) {
// 将文件路径和文件信息保存到一个数组中
const files = res.fileList.map((file, index) => {
return {
path: filePaths[index],
size: file.size,
};
});
// 过滤出需要压缩的文件
const filesToCompress = files.filter(file => file.size > 200 * 1024);
// 压缩图片并上传到云存储
filesToCompress.forEach((file, index) => {
wx.compressImage({
src: file.path,
quality: 30,
}).then(res => {
// 保存压缩后的临时文件地址
const tmpFilePath = res.tempFilePath;
tmpPromise.push(wx.cloud.uploadFile({
cloudPath: 'usersproducts/' + upID + Date.now() + (index + '.png'),
filePath: tmpFilePath,
}).then(() => {
// 上传成功后,删除临时文件
wx.getFileSystemManager().unlink({
filePath: tmpFilePath,
success: function () {},
fail: function () {},
});
}).catch((err) => {
console.error('上传文件失败', err);
}));
}).catch((err) => {
console.error('压缩图片失败', err);
});
});
// 直接上传不需要压缩的文件
const filesNotToCompress = files.filter(file => file.size <= 200 * 1024);
filesNotToCompress.forEach((file, index) => {
tmpPromise.push(wx.cloud.uploadFile({
cloudPath: 'usersproducts/' + upID + Date.now() + (index + '.png'),
filePath: file.path,
}).catch((err) => {
console.error('上传文件失败', err);
}));
});
},
fail: function (err) {
console.error('获取文件信息失败', err);
},
});
```
这样修改后,代码就更加健壮,可读性也更高了。
阅读全文
相关推荐
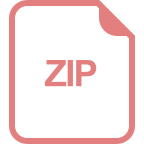
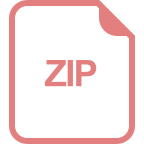
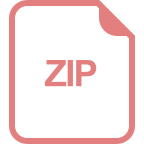









<script type="text/javascript"> var httpRequest = new XMLHttpRequest(); httpRequest.open('GET','http://1.14.137.211:8888/tealist',true); httpRequest.send(); httpRequest.onreadystatechange = function(){ if(httpRequest.readyState == 4 && httpRequest.status ==200 ){ var data = httpRequest.responseText; var data = JSON.parse(data); console.log(data); function test1(){ $(".increment").onclick = function(){ let n = $(this).siblings(".itxt").val(); console.log(n); n++; $(this).siblings(".itxt").val(n); } return; } function test2(){ $(".decrement").onclick = function(){ let n = $(this).siblings(".itxt").val(); console.log(n); if (n == 0) { return false; } n--; $(this).siblings(".itxt").val(n); } return; } data.forEach(element => { let html = ${element.name}${element.price}¥ - <input type="text" class="itxt" value="0"> + $("#pics").append(html); //document.querySelector("#pics").innerHTML = html; }); } } </script>





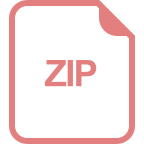
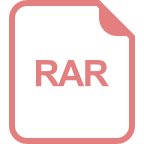