(只有php页面和文件夹)编写 PHP 页面实现一个留言板,包括留言者的 id(可以是网名),时间, 主题,正文和附件;另外设计一个页面对留言进行列表,以表格的形式展示留言的简介;当点击一条信息后,显示该留言的详细信息(可以查看附件)
时间: 2023-11-21 11:05:31 浏览: 202
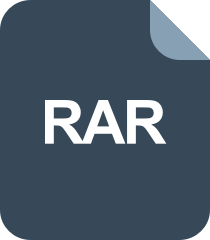
一个php做的留言板
好的,我可以为您提供一些基本的代码框架和思路。
首先,我们需要创建一个名为“message_board”的数据库,并在其中创建一个名为“messages”的表,该表包括以下字段:
- id:留言的唯一标识符,自增长整数类型;
- user_id:留言者的id,字符串类型;
- time:留言时间,日期时间类型;
- subject:留言主题,字符串类型;
- message:留言正文,文本类型;
- attachment:附件,字符串类型(保存附件的文件名)。
接下来,我们需要创建两个 PHP 页面:一个用于添加新留言,另一个用于显示留言列表和详细信息。
1. 添加新留言页面
在此页面中,我们将创建一个表单,以便用户输入其留言信息。在用户提交表单时,我们将使用 PHP 将其信息插入到数据库中。
```php
<!DOCTYPE html>
<html>
<head>
<title>Add New Message</title>
</head>
<body>
<h1>Add New Message</h1>
<form action="add_message.php" method="POST" enctype="multipart/form-data">
<label for="user_id">User ID:</label>
<input type="text" name="user_id" required><br>
<label for="subject">Subject:</label>
<input type="text" name="subject" required><br>
<label for="message">Message:</label>
<textarea name="message" rows="5" required></textarea><br>
<label for="attachment">Attachment:</label>
<input type="file" name="attachment"><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
```
在此表单中,我们使用了“enctype”属性,以便支持上传附件。当用户提交表单时,我们将使用“add_message.php”页面处理其信息。
2. 处理新留言页面
在此页面中,我们将接收来自添加新留言表单的信息,并将其插入到数据库中。如果用户上传了附件,则我们需要将其保存到服务器上的一个文件夹中,并在数据库中保存附件的文件名。
```php
<?php
// 连接到数据库
$conn = mysqli_connect("localhost", "username", "password", "message_board");
// 检查连接是否成功
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
// 获取表单数据
$user_id = $_POST['user_id'];
$subject = $_POST['subject'];
$message = $_POST['message'];
// 上传附件
$attachment = "";
if (!empty($_FILES['attachment'])) {
$target_dir = "uploads/"; // 保存附件的文件夹
$target_file = $target_dir . basename($_FILES["attachment"]["name"]);
move_uploaded_file($_FILES["attachment"]["tmp_name"], $target_file);
$attachment = basename($_FILES["attachment"]["name"]);
}
// 将留言插入到数据库中
$sql = "INSERT INTO messages (user_id, time, subject, message, attachment) VALUES ('$user_id', NOW(), '$subject', '$message', '$attachment')";
if (mysqli_query($conn, $sql)) {
echo "New message added successfully!";
} else {
echo "Error: " . $sql . "<br>" . mysqli_error($conn);
}
// 关闭数据库连接
mysqli_close($conn);
?>
```
3. 显示留言列表和详细信息页面
在此页面中,我们将从数据库中检索所有留言,并将其以表格的形式显示出来。当用户单击留言标题时,我们将显示该留言的详细信息,在此页面上还将显示该留言的附件(如果有)。
```php
<!DOCTYPE html>
<html>
<head>
<title>Message Board</title>
</head>
<body>
<h1>Message Board</h1>
<?php
// 连接到数据库
$conn = mysqli_connect("localhost", "username", "password", "message_board");
// 检查连接是否成功
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
// 获取所有留言
$sql = "SELECT * FROM messages";
$result = mysqli_query($conn, $sql);
if (mysqli_num_rows($result) > 0) {
// 显示留言列表
echo "<table border='1'>";
echo "<tr><th>ID</th><th>User ID</th><th>Time</th><th>Subject</th><th>Attachment</th></tr>";
while($row = mysqli_fetch_assoc($result)) {
echo "<tr>";
echo "<td>" . $row["id"] . "</td>";
echo "<td>" . $row["user_id"] . "</td>";
echo "<td>" . $row["time"] . "</td>";
echo "<td><a href='message_detail.php?id=" . $row["id"] . "'>" . $row["subject"] . "</a></td>";
echo "<td>" . $row["attachment"] . "</td>";
echo "</tr>";
}
echo "</table>";
} else {
echo "No messages found.";
}
// 关闭数据库连接
mysqli_close($conn);
?>
</body>
</html>
```
在此页面中,我们使用了“message_detail.php”页面来显示单个留言的详细信息。我们将使用 URL 参数(即“id”)将要显示的留言的 ID 传递给该页面。
4. 显示留言详细信息页面
在此页面中,我们将显示单个留言的详细信息,包括其附件(如果有)。
```php
<!DOCTYPE html>
<html>
<head>
<title>Message Detail</title>
</head>
<body>
<h1>Message Detail</h1>
<?php
// 连接到数据库
$conn = mysqli_connect("localhost", "username", "password", "message_board");
// 检查连接是否成功
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
// 获取留言的 ID
$id = $_GET['id'];
// 获取留言详细信息
$sql = "SELECT * FROM messages WHERE id=$id";
$result = mysqli_query($conn, $sql);
if (mysqli_num_rows($result) > 0) {
$row = mysqli_fetch_assoc($result);
echo "<p><strong>User ID:</strong> " . $row["user_id"] . "</p>";
echo "<p><strong>Time:</strong> " . $row["time"] . "</p>";
echo "<p><strong>Subject:</strong> " . $row["subject"] . "</p>";
echo "<p><strong>Message:</strong> " . $row["message"] . "</p>";
if (!empty($row["attachment"])) {
echo "<p><strong>Attachment:</strong> <a href='uploads/" . $row["attachment"] . "'>" . $row["attachment"] . "</a></p>";
}
} else {
echo "Message not found.";
}
// 关闭数据库连接
mysqli_close($conn);
?>
</body>
</html>
```
以上是基本的代码框架和思路,您可以根据自己的需要进行更改和扩展。
阅读全文
相关推荐
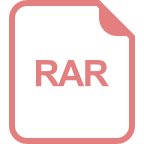

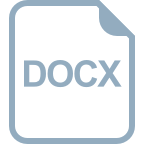
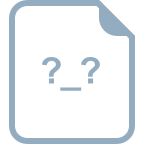
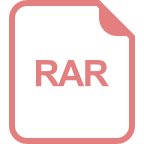
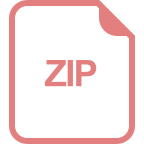
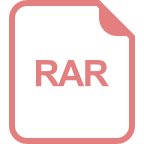
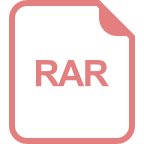
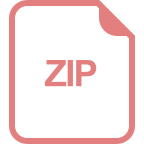
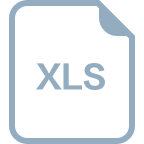
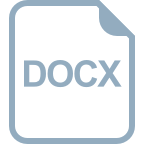
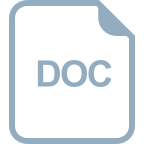


