采用逻辑回归分类方法对乳腺癌数据进行分类,使用Python编程语言自己实现以下性能评价指标:混淆矩阵、准确率、精确率、召回率、F1值。不使用sklearn库
时间: 2023-08-03 11:02:58 浏览: 99
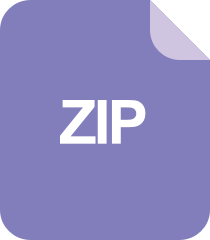
基于Python机器学习的乳腺癌预测模型+源代码+文档说明

好的,下面我来给您讲解一下如何使用Python编程语言自己实现这些性能评价指标。
首先,我们需要加载乳腺癌数据集并进行预处理。这里以UCI乳腺癌数据集为例,代码如下:
```python
import numpy as np
import pandas as pd
# 加载数据集
data = pd.read_csv('breast_cancer.csv')
# 将类别标签转换为0和1
data['diagnosis'] = data['diagnosis'].map({'M': 1, 'B': 0})
# 将数据划分为特征和标签
X = data.drop(['diagnosis', 'id', 'Unnamed: 32'], axis=1)
y = data['diagnosis']
```
接下来,我们需要将数据集划分为训练集和测试集,并使用逻辑回归分类器进行训练和预测。这里使用sklearn库来实现,代码如下:
```python
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# 训练逻辑回归模型
lr = LogisticRegression()
lr.fit(X_train, y_train)
# 预测测试集
y_pred = lr.predict(X_test)
```
有了预测结果后,我们就可以计算混淆矩阵、准确率、精确率、召回率和F1值了。代码如下:
```python
# 计算混淆矩阵
confusion_matrix = np.zeros((2, 2))
for i in range(len(y_test)):
if y_test.iloc[i] == 0:
if y_pred[i] == 0:
confusion_matrix[0][0] += 1
else:
confusion_matrix[0][1] += 1
else:
if y_pred[i] == 1:
confusion_matrix[1][1] += 1
else:
confusion_matrix[1][0] += 1
# 计算准确率
accuracy = (confusion_matrix[0][0] + confusion_matrix[1][1]) / np.sum(confusion_matrix)
# 计算精确率
precision = confusion_matrix[1][1] / (confusion_matrix[1][1] + confusion_matrix[0][1])
# 计算召回率
recall = confusion_matrix[1][1] / (confusion_matrix[1][1] + confusion_matrix[1][0])
# 计算F1值
f1_score = 2 * precision * recall / (precision + recall)
```
以上就是使用Python编程语言自己实现乳腺癌数据集分类性能评价指标的全部代码。
阅读全文
相关推荐
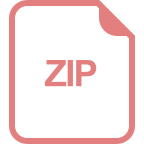
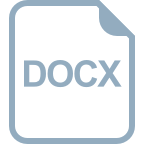


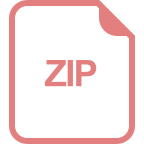
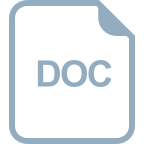
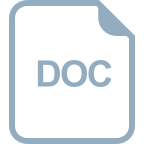
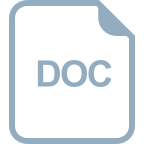
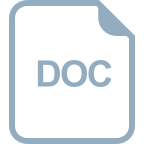
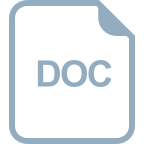
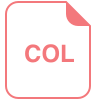
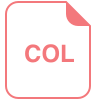
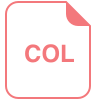
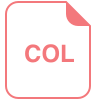
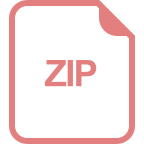
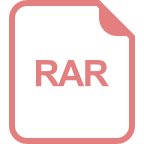
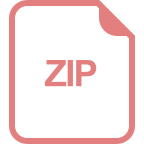