用python,百度智能云和tk做内容审核:视频
时间: 2024-04-02 20:35:33 浏览: 175
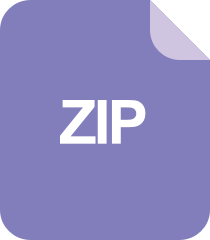
基于百度AI的内容审核程序.zip
好的,针对你的问题,我们可以使用Python,百度智能云的内容审核API和Tkinter库来实现一个简单的GUI界面,用于上传和审核视频文件。具体步骤如下:
1. 首先,我们需要在百度智能云控制台申请内容审核服务,并获取相应的API Key和Secret Key。
2. 然后,在Python中安装百度智能云Python SDK,并使用API Key和Secret Key进行身份验证。
3. 接下来,我们可以使用Tkinter库创建一个GUI界面,用于上传和审核视频文件。
4. 在GUI界面中,我们可以添加一个“上传”按钮,用于选择待审核的视频文件,并调用审核接口进行审核。
5. 审核完成后,我们可以在GUI界面中显示审核结果,并根据审核结果来处理视频文件。
下面是一个简单的示例代码,用于实现视频内容审核的GUI界面:
```
import tkinter as tk
from tkinter import filedialog
from aip import AipContentCensor
# 设置百度智能云应用信息
APP_ID = 'your_app_id'
API_KEY = 'your_api_key'
SECRET_KEY = 'your_secret_key'
# 初始化审核客户端
client = AipContentCensor(APP_ID, API_KEY, SECRET_KEY)
# 设置审核参数
options = {
'type': 'video',
'scenes': ['antiporn', 'terror'],
}
# 创建GUI界面
root = tk.Tk()
root.title('视频内容审核')
# 定义选择文件的函数
def choose_file():
file_path = filedialog.askopenfilename()
file_entry.delete(0, tk.END)
file_entry.insert(0, file_path)
# 定义审核文件的函数
def audit_file():
file_path = file_entry.get()
with open(file_path, 'rb') as f:
video_data = f.read()
result = client.videoCensor(video_data, options)
if result['conclusion'] == '合规':
result_label.config(text='审核通过!')
else:
result_label.config(text='审核未通过,审核结果为:' + result['conclusion'])
# 创建GUI界面的组件
file_label = tk.Label(root, text='请选择待审核的视频文件:')
file_entry = tk.Entry(root, width=50)
file_button = tk.Button(root, text='选择文件', command=choose_file)
audit_button = tk.Button(root, text='开始审核', command=audit_file)
result_label = tk.Label(root, text='')
# 将组件添加到GUI界面中
file_label.pack()
file_entry.pack()
file_button.pack()
audit_button.pack()
result_label.pack()
# 启动GUI界面
root.mainloop()
```
需要注意的是,以上示例代码仅供参考,实际使用时还需要根据具体情况进行适当的修改和调整。另外,为了保证审核结果的准确性和可靠性,建议在使用时仔细阅读官方文档,并按照文档中的要求进行参数设置和调用方式。
阅读全文
相关推荐
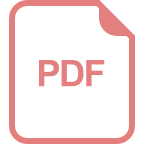
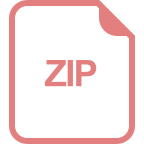
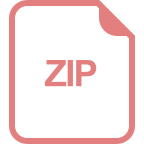
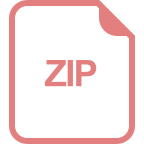
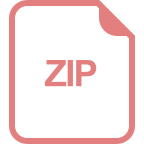
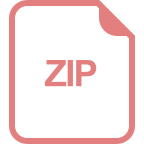
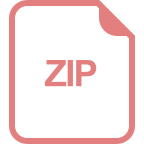
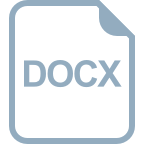
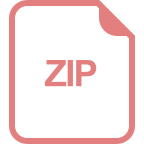
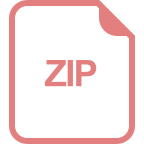
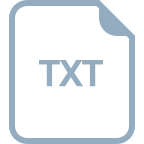
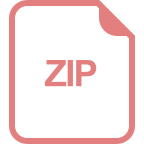
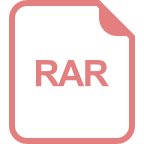
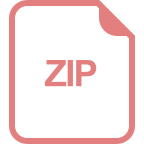
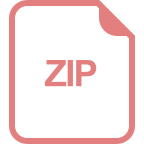
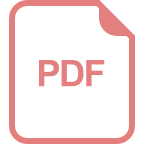