design a model using pytorch embedding
时间: 2024-05-13 10:20:42 浏览: 119
Sure, here's an example of how you can design a PyTorch model using embeddings:
```
import torch
import torch.nn as nn
class EmbeddingModel(nn.Module):
def __init__(self, vocab_size, embedding_dim, hidden_size, num_classes):
super(EmbeddingModel, self).__init__()
self.embedding = nn.Embedding(vocab_size, embedding_dim)
self.rnn = nn.LSTM(embedding_dim, hidden_size, batch_first=True)
self.fc = nn.Linear(hidden_size, num_classes)
def forward(self, inputs):
# inputs shape: (batch_size, seq_len)
embedded = self.embedding(inputs)
# embedded shape: (batch_size, seq_len, embedding_dim)
output, _ = self.rnn(embedded)
# output shape: (batch_size, seq_len, hidden_size)
logits = self.fc(output[:, -1, :])
# logits shape: (batch_size, num_classes)
return logits
```
In this example, we're creating a model that takes in sequences of integers (representing words in a sentence) and outputs a classification.
The `EmbeddingModel` class inherits from `nn.Module` and defines three layers:
1. An `Embedding` layer that creates a learned embedding for each word in the vocabulary. The `vocab_size` parameter specifies the number of unique words in the vocabulary, and `embedding_dim` specifies the size of the learned embeddings.
2. An `LSTM` layer that takes the embedded input sequences and outputs a sequence of hidden states. The `hidden_size` parameter specifies the number of hidden units in the LSTM.
3. A fully connected `Linear` layer that takes the final hidden state of the LSTM and produces the output logits. `num_classes` specifies the number of classes we're trying to classify.
In the `forward` method, we first pass the input sequences through the embedding layer to get the learned embeddings. Then we pass the embedded sequences through the LSTM layer to get a sequence of hidden states. Finally, we take the last hidden state (corresponding to the end of the sequence) and pass it through the fully connected layer to get the final logits.
Note that we're using the `batch_first=True` parameter in the LSTM layer so that the input and output shapes are `(batch_size, seq_len, embedding_dim)` and `(batch_size, seq_len, hidden_size)` instead of `(seq_len, batch_size, embedding_dim)` and `(seq_len, batch_size, hidden_size)`. This is just a matter of personal preference, but it can make the code easier to read and write.
阅读全文
相关推荐
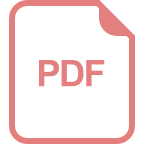


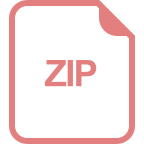
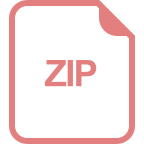
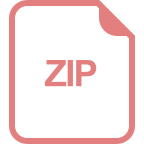
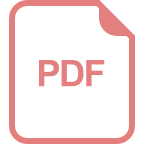
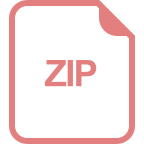
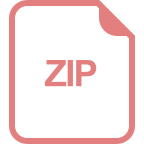
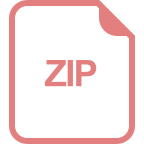
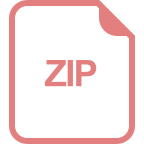
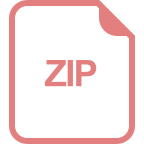
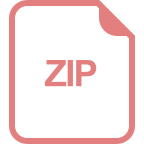
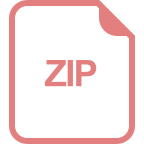
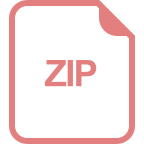
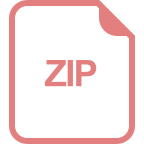
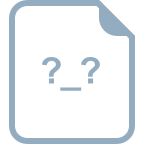